Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial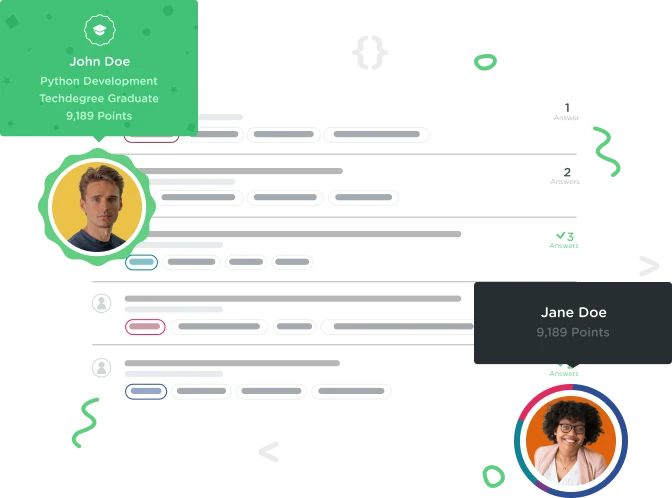
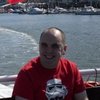
Sean Flanagan
33,236 PointsHow do I get my output to be like Jason's?
address.rb:
class Address
attr_accessor :kind, :street_1, :street_2, :city, :state, :postal_code
def to_s(format = "short")
address = " "
case format
when "long"
address += street_1 + "\n"
address += street_2 + "\n" if !street_2.nil?
address += "#{city}, #{state} #{postal_code}"
when "short"
address += "#{kind}: "
address += street_1
if street_2
address += " " + street_2
end
address += ", #{city}, #{state}, #{postal_code}"
end
address
end
end
phone_number.rb:
class PhoneNumber
attr_accessor :kind, :number
def to_s
"#{kind}: #{number}"
end
end
contact.rb:
require "./phone_number"
require "./address"
class Contact
attr_writer :forename, :middle_name, :surname
attr_reader :phone_numbers, :addresses
def initialize
@phone_numbers = []
@addresses = []
end
def add_phone_number(kind, number)
phone_number = PhoneNumber.new
phone_number.kind = kind
phone_number.number = number
phone_numbers.push(phone_number)
end
def add_address(kind, street_1, street_2, city, state, postal_code)
address = Address.new
address.kind = kind
address.street_1 = street_1
address.street_2 = street_2
address.city = city
address.state = state
address.postal_code = postal_code
addresses.push(address)
end
def forename
@forename
end
def middle_name
@middle_name
end
def surname
@surname
end
def forename_first
forename + " " + surname
end
def surname_first
surname_first = surname
surname_first += ", "
surname_first += forename
if !middle_name.nil?
surname_first += " "
surname_first += middle_name.slice(0, 1)
surname_first += "."
end
surname_first
end
def full_name
full_name = forename
if !middle_name.nil?
full_name += " "
full_name += middle_name
end
full_name += ' '
full_name += surname
full_name
end
def to_s(format = "full_name")
case format
when "full_name"
full_name
when "surname_first"
surname_first
when "first"
forename
when "last"
surname
else
surname_first
end
end
def print_phone_numbers
puts "Phone Numbers"
phone_numbers.each { |phone_number| puts phone_number }
end
def print_addresses
puts "Addresses"
addresses.each { |address| puts address.to_s("short") }
end
end
sean = Contact.new
sean.forename = "Sean"
sean.middle_name = "Michael"
sean.surname = "Flanagan"
sean.add_phone_number("Home", "123-456-7890")
sean.add_phone_number("Work", "456-789-0123")
sean.add_address("Home", "123 Main St.", "", "Portland", "OR", "12345")
puts sean.to_s("full_name")
sean.print_phone_numbers
sean.print_addresses
address_book.rb:
require "./contact"
class AddressBook
attr_reader :contacts
def initialize
@contacts = []
end
def print_contact_list
puts "Contact List"
contacts.each do |contact|
puts contact.to_s("surname_first")
end
end
end
address_book = AddressBook.new
sean = Contact.new
sean.forename = "Sean"
sean.surname = "Flanagan"
sean.add_phone_number("Home", "123-456-7890")
sean.add_phone_number("Work", "456-789-0123")
sean.add_address("Home", "123 Main St.", "", "Portland", "OR", "12345")
marcia = Contact.new
marcia.forename = "Marcia"
marcia.surname = "Scott"
marcia.add_phone_number("Home", "222-222-2222")
marcia.add_address("Home", "222 Two Lane", "", "Portland", "OR", "12345")
address_book.contacts.push(sean)
address_book.contacts.push(marcia)
address_book.print_contact_list
I'd appreciate any help.
Ken jones
5,394 PointsKen jones
5,394 PointsAt the bottom of your contact.rb file you have this.....
You need to remove that from the file as those commands will run when you require this file from within address_book.rb. Thats Why your output will look different to Jason's when you run address_book.rb from the console.