Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial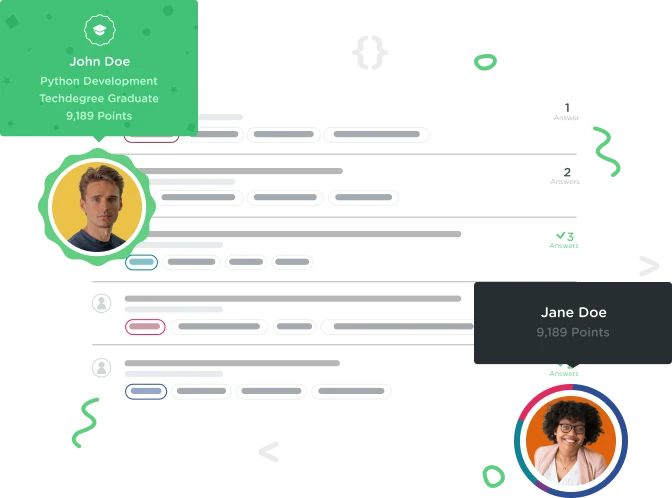

Shawn Vogler
564 PointsHow do I return a remainder inside a method?
"Inside the "mod" method, write the necessary code to return the remainder of "a" when divided by "b." Hint: remember the "%" operator!"
So here's what I've got so far:
def mod (a, b)
puts a / b
return a%, b%
end
mod (10%), (2%)
It's been so long using the "%" operator. Also, I'm pretty confused about the "return" method.
2 Answers

Shawn Vogler
564 PointsI feel like a dummy. All I had to was change it from puts to return. It looks like this.
def mod (a, b)
puts "#{a} divided by #{b} = "
puts a / b
puts "The remainder of #{a} divided by #{b} is : "
return a % b
end
mod (10), (7)

Vlad Filiucov
10,665 PointsHi,
You wrote to much. One line is enough. You don't need return key word in ruby. Last thing method does will be returned so you can get rid of it. You can get rid of puts as well for the same reason as above. And in your method you are trying to display same string 3 times. And also when you are calling your mod method you should pass all arguments inside the brackets, and separated by comma. Also don't leave spaces between method name and argument bracket. So at the end of the day it should look something like this
def mod(a, b)
"The remainder of #{a} divided by #{b} is : #{a%b}"
end
mod(10, 7)
Shawn Vogler
564 PointsShawn Vogler
564 PointsHere's what I've updated my code to look like:
When I look at the preview it is showing this: "10 divided by 7 = 1 The remainder of 10 divided by 7 is : 3" It's outputting the remainder obviously? What am I missing here?