Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial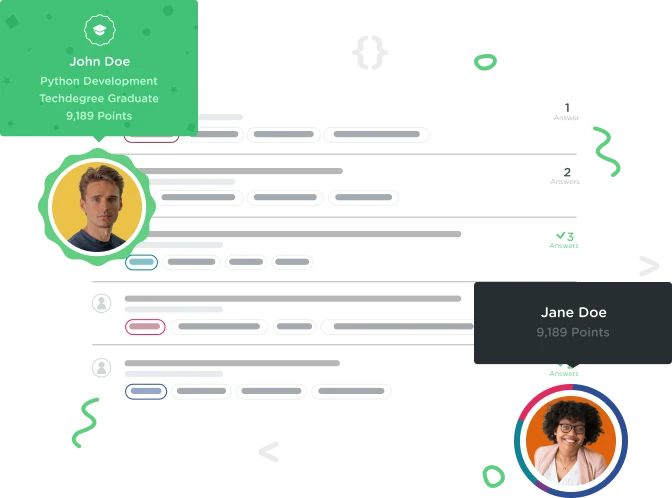

Unsubscribed User
3,120 PointsHow do I turn arguments into floats
How do I turn arguments into floats? It tells me it can't float a string so I tried turning it into an int.
def add (a, b):
sum = int(float(a + b))
return sum
5 Answers

Ryan S
27,276 PointsHi Ezekiel,
You are on the right track with the float() function, however you need to convert each argument separately.
>>> float(a) + float(b)
Good luck,
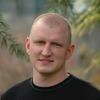
Chris Howell
Python Web Development Techdegree Graduate 49,702 PointsTo kind of build onto @Ryan S answer.
You can only pass String version of numbers or floats into float. If you pass Letter Characters it will raise ValueError.
EXAMPLES
So in the Python Shell if I do this:
Passing a Number as a String
>>> float("3")
3.0
Passing a String with Characters
>>> float("three")
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: could not convert string to float: 'three'
Passing a Float as a String
>>> float("2.0")
2.0
Passing an Integer
>>> float(2)
2.0
NOTE: Dont type >>>. I am immitating the look of Python Shell with my commands.
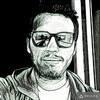
Felipe Balbino
Courses Plus Student 686 PointsChris Howell how come it works when I'm trying out on my machine? (I'm running 2.7) Is that something that was changed in Python 3?
Sry for dropping down like that on your post, Ezekiel Wootton :P
def add(x,y):
return float(x + y)
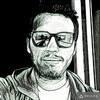
Felipe Balbino
Courses Plus Student 686 PointsTks a lot, Ryan S . I got it now! :)

Ryan S
27,276 PointsHi Felipe,
You can evaluate expressions inside the float function, so yes it will work in both Python 2 and 3. But keep in mind that this is fine as long as the arguments are guaranteed to be integers.
This challenge wants you to handle the potential case that the arguments are numeric strings because the expression inside the function will evaluate differently if that is the case.
So for example, integers will work as you'd expect
>>> a = 5
>>> b = 6
>>> float(a + b)
11.0
But now try them as numeric strings:
>>> a = "5"
>>> b = "6"
>>> float(a + b)
56.0
The strings will concatenate first, then convert to a float, which is why it won't pass the challenge.
Hope this clears things up.
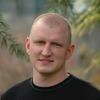
Chris Howell
Python Web Development Techdegree Graduate 49,702 PointsChanged from Comment to an Answer :)