Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial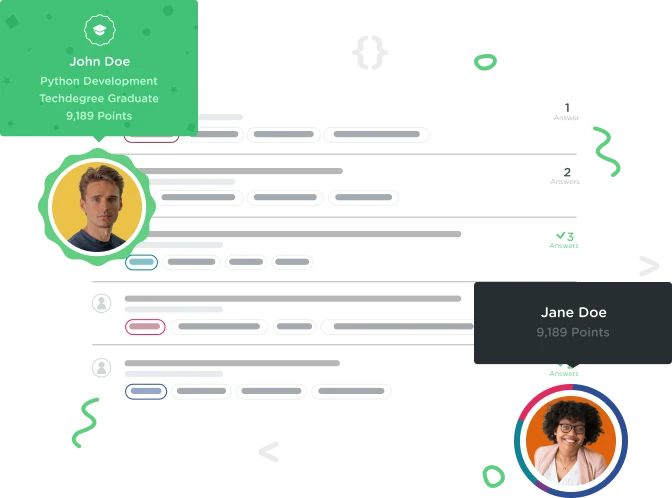

Dan Nelson
846 Pointshow do you make a list of lists
I have this working, but it does not seem to be the list of list that is wanted. here is my code. I did get the first 2 parts of the challenge, but cannot find a format of list of lists that is acceptable.
def stats(my_dict): name_list = [] count_list = [] list_of_list = [] num_classes = 0
for name in my_dict: count = 0 name_list.append(name) for classes in my_dict[name]: count = count+1 str_count = str(count) count_list.append(str_count) list_of_list = [name_list, count_list]
return list_of_list
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
def most_classes(my_dict):
max_name = ''
max_count = 0
for name in my_dict:
count =0
for classes in my_dict[name]:
count = count+1
if count>max_count:
max_name = name
max_count = count
return max_name
def num_teachers(my_dict):
count = 0
max_count = 0
for name in my_dict:
count = count+1
max_count = count
return max_count
def stats(my_dict):
name_list = []
count_list = []
list_of_list = []
num_classes = 0
for name in my_dict:
count = 0
name_list.append(name)
for classes in my_dict[name]:
count = count+1
str_count = str(count)
count_list.append(str_count)
list_of_list = [name_list, count_list]
print (list_of_list)
return list_of_list
2 Answers

Keerthi Suria Kumar Arumugam
4,585 PointsThe problem is because you are defining your list of lists as : list_of_list = [name_list, count_list]
How do you append an item to a list? my_list.append(new_item)
How do you append a list to a list? my_list.append(new_list)
So if you append your list_of_list for every teacher's name, instead of defining it as list_of_list = [name_list, count_list], you will get your expected output
This code works fine now :
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
def most_classes(my_dict):
max_name = ''
max_count = 0
for name in my_dict:
count =0
for classes in my_dict[name]:
count = count+1
if count>max_count:
max_name = name
max_count = count
return max_name
def num_teachers(my_dict):
count = 0
max_count = 0
for name in my_dict:
count = count+1
max_count = count
return max_count
def stats(my_dict):
name_list = []
count_list = []
list_of_list = []
num_classes = 0
for name in my_dict:
count = 0
for classes in my_dict[name]:
count = count+1
list_of_list.append([name, count])
return list_of_list

Keerthi Suria Kumar Arumugam
4,585 PointsIf you use list_of_list.append((name, count)), the component (name, count) is a tuple, you need to enclose it in square brackets to make that a list.
Dan Nelson
846 PointsDan Nelson
846 PointsThanks for the help. I finally went back and tried this again. I think the wording on the question is confusing as I tried many ways to make this list and was not able to get that what was desired was a list pair of Name and class count. I interpreted this as a list of name and a list of class counts. I don't think I understand what the <> mean. Where was this explained.
I also tried to make a list of list, but used (what I now know as a tupple) and python took it, but it did not give the correct answer. This left me confused: I used list_of_list.append((name, count)) in the same place and it gives a very close answer, but with () not []. This was very confusing until the tupple discussion in the next section.
I want this to be constructive feedback and I hope this can be made more clear.