Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial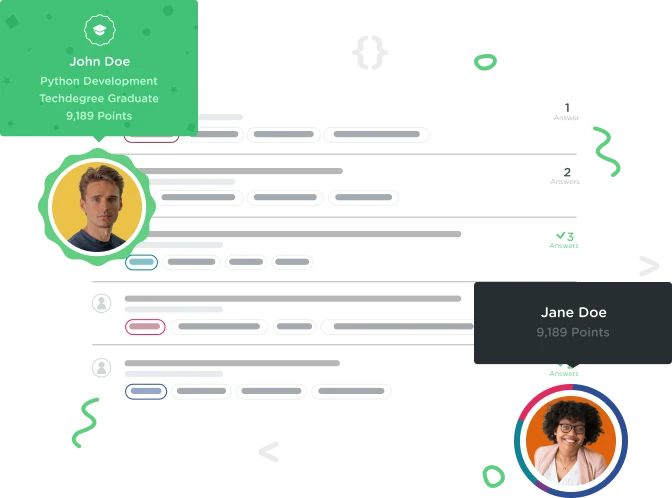
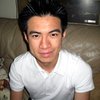
jason chan
31,009 PointsHow does static public work for php?
<?php
class Address {
static public $valid_address_types = array(
1 => 'Residence',
2 => 'Business',
3 => 'Park',
);
public $street_address_1;
public $street_address_2;
// Name of the city
public $city_name;
// name of the subdivision.
public $subdivision_name;
// Postal code
protected $_postal_code;
// name of the country
public $country_name;
// primary key of an address
protected $_address_id;
// when the record was created and last updated
protected $_time_created;
protected $_time_updated;
// magic get; returns mixed
function __construct($data = array()) {
$this->_time_created = time();
// ensure the property can be populated
if (!is_array($data)) {
trigger_error('Unable to construct address with a ' . get_class($name));
}
// if there is at least one value, populate the address with it.
if (count($data) > 0) {
foreach ($data as $name => $value) {
// special case for protected properties
if (in_array($name, array( 'time_created', 'time_updated'))) {
$name = '_' . $name;
}
$this->$name = $value;
}
}
}
function __get($name) {
// Postal code lookup if unset.
if (!$this->_postal_code) {
$this->_postal_code = $this->_postal_code_guess();
}
// attempt to return a protected property by name.
$protected_property_name = '_' . $name;
if (property_exists($this, $protected_property_name)) {
return $this->$protected_property_name;
}
trigger_error('Undefinited property via __get ' . $name);
return NULL;
}
protected function _postal_code_guess() {
return 'LOOKUP';
}
function __set($name, $value) {
// Allow anything to set the postal code.
if ('postal_code' == $name) {
$this->$name = $value;
return;
}
// unable access property trigger error
trigger_error('Undefined or unallowed property via __set(): ' . $name);
}
// display an addres in html
function display () {
$output = '';
// street address
$output .= $this->street_address_1;
if ($this->street_address_2) {
$output .= '<br />' . $this->street_address_2;
}
// City, subdivision Postal.
$output .= '<br/>' ;
$output .= $this->city_name . ' , ' . $this->subdivision_name;
$output .= ' ' . $this->postal_code;
$output .= '<br/>';
$output .= $this->country_name;
return $output;
}
function __toString() {
return $this->display();
}
}
My other file
<?php
require 'class.Address.inc';
?>
<h2>Instantiating address</h2><br>
<h2>Empty address</h2>
<?php
$address = new Address;
echo '<tt><pre>' . var_export($address, TRUE) . '</pre></tt>';
?>
<br>
<?php
$address->street_address_1 = '555 fake st';
$address->city_name = "Some City";
$address->subdivision_name = "State";
$address->postal_code = "90000";
$address->country_name = "USA";
echo '<tt><pre>' . var_export($address, TRUE) . '</pre></tt>';
?>
<h2>Display Address...</h2>
<?php
echo $address->display();
?>
<h3>testing magic __get and __set</h3>
<?php
unset($address->postal_code);
echo $address->display();
?>
<h2>_construct with an array</h2>
<?php
$address_2 = new Address(array(
'street_address_1' => '123 place',
'city_name' => 'Villageland',
'subdivision_name' => 'Region',
'postal_code' => '99999',
'country_name' => 'canada',
));
echo $address_2->display();
?>
<h2>address __toString</h2>
<?php
echo $address_2;
?>
<h2>Displaying Address Types..</h2>
<?php
// why did it become :: this vs ->
echo "<tt><pre> " . var_export(Address::$valid_address_types, TRUE) . " </tt></pre>";
?>
So why does static public for calling a method use :: vs -> ?
1 Answer

Petros Sordinas
16,181 PointsHi Jason,
To put it shortly, because PHP works that way :)
A static method or property can be accessed without instantiating the class. Because of this, the $this pseudo variable can't be used to access the method or property and it is referenced with the double colon :: and the name of the class before it.
If you were to access a static within the class, you would use self:: or if you to access a static property of a parent class, you would use parent::
Here is the php documentation on this http://php.net/manual/en/language.oop5.static.php
jason chan
31,009 Pointsjason chan
31,009 PointsI was hoping for why reason. Now, it's just it's because it is answer thanks anyway.