Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial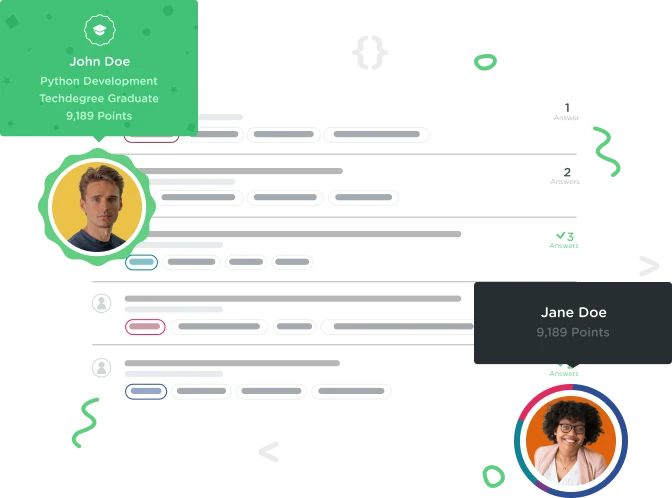
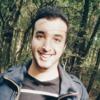
baderbouta
7,804 PointsHow does this code look Would love some feedback?
Hey guys (and girls),
I would love some feedback on this piece of code I wrote, It was kinda hard actually but I think it works!
//Takes any number from a user and parses it into a 'integer' data-type
var firstUserNumbInput = parseInt(prompt("Hello, can you please give me a number?"));
//Takes any number from a user for the second time and parses it into a 'integer' data-type
var secondUserNumbInput = parseInt(prompt("Cool thank you for giving me the number " + firstUserNumbInput + ", I would now like you to give me another random number if you'd like!"));
//Alerts user of the their chosen number
alert("Thanks! your number of choice is " + firstUserNumbInput + ", and " + secondUserNumbInput + ". I will now generate a random number from your number");
//Generates a random number based on the user input and uses a 'Math.round' method to make it into a round number
alert("The number that I've generated from your input is " + Math.round(Math.random() * secondUserNumbInput + firstUserNumbInput));
4 Answers
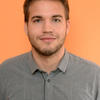
Adam Beer
11,314 PointsLooks good! Bravo! Maybe I would change the last alert dialog to document.write(). Like this:
document.write("<h2>The number that I've generated from your input is " + Math.round(Math.random() * secondUserNumbInput + firstUserNumbInput) + "</h2>");
But that is your decision. Happy coding!

kevin hudson
Courses Plus Student 11,987 PointsYour code does not follow the extra credit instructions so it is not the right answer. I am currently trying to solve this myself.
so if I put 8 as the first number and 10 and the second - the code ads 8 + 10 which equals 18 then Math.random from zero to 18 which can be outside the values the user sets -
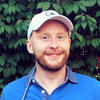
Michael Kilcorse
Full Stack JavaScript Techdegree Graduate 17,643 PointsSo what Kevin is talking about gave me a lot of grief for a really long time. I wanted to make it work for any two positive integers the user would input. I finally figured it out after about three hours of trying everything I could think of, I finally figured it out!
var num1;
var num2;
var rand;
var limit;
num1 = parseInt(prompt("Please Enter your first number: "));
num2 = parseInt(prompt("Please Enter your second number: "));
limit = (num2 - num1)/2;
rand = Math.abs(Math.round(Math.random() * (num2 - num1)) + num1);
alert("Your random number between " + num1 + " and " + num2 + " is " + rand + "!");
by subtracting the inputs, you create a new limit for Math.random. examples: 1 through 5 will be taken as 5-1=4 that 4 is the new limit that is multiplied by the random number, but then by adding the first number to that, you guarantee the full range of possibilities. 4+1=5
This works on large numbers as well: 56 through 89 89-56 = 33 Math.random() * 33 even if Math.random gave you 33 because that is what's set as the maximum value, you then add 56 to it, returning your maximum value back up to 89
(sorry if this is a hamfisted way of explaining this, lol, I don;t know math terms and I'm new to coding)
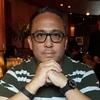
hapaman
Full Stack JavaScript Techdegree Student 3,026 PointsOkay, I came up with two ways to do the basic project, and made an attempt at the extra credit one, by using what I saw on MDN. Any and all feedback is appreciated.
//Rounds down the random number
var userNum = prompt("I will generate a random number between 1 and a maximum number that you specify. Please enter the maximum number.");
randomNum = Math.floor((Math.random() * userNum) + 1);
document.write("<h2>Your random number is: " + randomNum + "</h2>");
// Parses the random number
var userNum = prompt("I will generate a random number between 1 and a maximum number that you specify. Please enter the maximum number.");
randomNum = parseInt(Math.random() * userNum) + 1;
document.write("<h2>Your random number is: " + randomNum + "</h2>");
//Extra credit task - inclusive range on low and high number
function getRandomNum(min, max) {
//console.log(min);
//console.log(max);
min = Math.ceil(min);
max = Math.floor(max);
//console.log(min);
//console.log(max);
var randomNum = Math.floor(Math.random() * (max - min + 1)) + min;
document.write("<h2>Your random number is: " + randomNum + "</h2>");
};
var lowNum = prompt("I will generate a random number a range that you specify \(example: 1 through 100\). Please the lower number for the range.");
var highNum = prompt("Thanks. Please enter the higher number for the range.");
alert("You entered the numbers " + lowNum + " and " + highNum + ".");
getRandomNum(lowNum,highNum);