Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial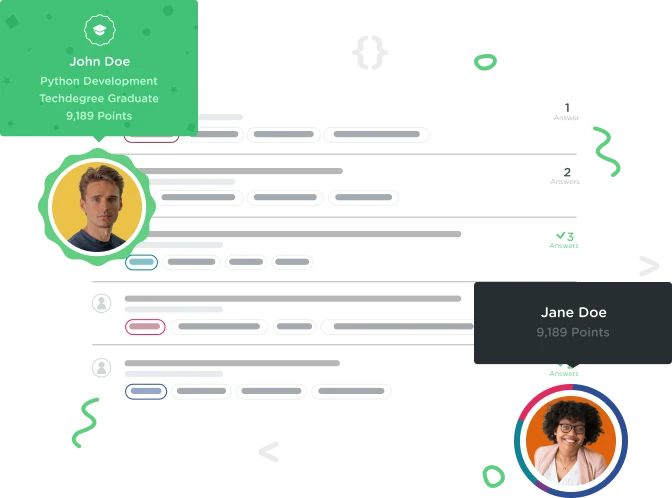
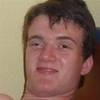
Anontawat Wiriyakijja
5,332 PointsHow does this code work?
This is my code:
var message = '';
var student;
var search;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function printStudentData(student) {
var data = '<h2>Student: ' + student.name + '</h2>';
data += '<p>Track: ' + student.track + '</p>';
data += '<p>Points: ' + student.points + '</p>';
data += '<p>Achievements: ' + student.achievements + '</p>';
return data;
}
while (true) {
search = prompt('Please type in student name or "quit" to exit the program.');
search.toLowerCase();
if (search === 'quit') {
break;
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (search === student.name.toLowerCase()) {
message = printStudentData(student);
print(message);
}
}
print("Sorry we could not find " + search + " in our database.");
}
I'd like to know that why did the print function at the bottom work after I typed "dave" in the prompt? Seemed like it didn't read the for loop? Please explain.
1 Answer
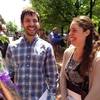
Mike D'Aguillo
5,475 PointsIt looks like you forgot another "}" after your break statement. Essentially, the while statement continues until after the print statement, so it's running the rest of the code.
I would suggest indenting your code when starting a code block. It makes it easier to see which statements exist within the same context. When you do this, it's easier to see that the if (search === 'quit') statement, the for loop, and the print statement all existed within the while statement.
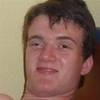
Anontawat Wiriyakijja
5,332 PointsThanks for your reply. I did indent my code but I just copied and pasted over from my workspace and I didn't preview before posted the comment.
The bracket for the while loop is under the last print function. Everything works just fine if I delete the last print function. I just want to know that why does it seem like skipping the for loop when I type in any word?
Chyno Deluxe
16,936 PointsChyno Deluxe
16,936 Points//Fixed Code Presentation How to post Code