Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial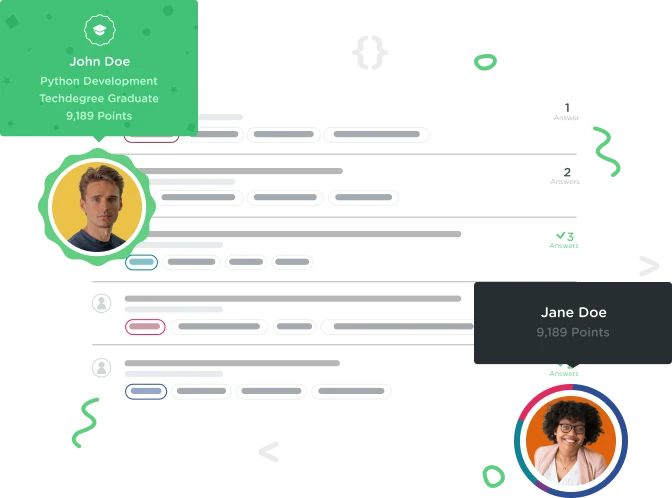

Greg Schudel
4,090 PointsHow is this wrong?
I don't understand how this is wrong. Should I just go back and review the whole section from the beginning again?
var secret = prompt("What is the secret password?");
do{ secret !== "sesame";
} while (prompt("What is the secret password?") );
document.write("You know the secret password. Welcome.");
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers

Daryl Carr
18,318 PointsA do, while loop basically tells JS to do something once and then run the while loop. It's normally set out like this;
do {
//Code goes here
} while (condition)
So basically when JS goes through this it will execute what ever is within the do { } and then it will check the while condition. If that condition is true then it will run what is in that do {code} and keep doing so until that while condition gives a false value.
In your case you want the do (} to run the prompt, so that the user is asked for the password. Their answer is stored in the secret variable. Now the while loop is tested. If the while condition is true, it will exit the loop otherwise it will run the do {} code again. So you want to do;
do {
var secret = prompt("What is the secret password?");
} while(secret !== "sesame")
This will keep the prompt appearing on the screen until the user enters the correct password --> "sesame"
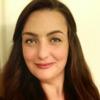
Jennifer Nordell
Treehouse TeacherIt's never a bad idea to review things you suspect you didn't really grasp! So I'd definitely recommend doing that if you feel completely lost. But before you do that, let's take a look at what your code does.
It asks for the password. The user puts in what they think the secret password is. And that guess is stored in a variable named secret. Now, no matter what they put in the first time it's still going to ask for the password again because this is a do loop. It means that this code will run at least once... always.
But there's no code inside your loop so it skips down to the while. And that's where it asks for the password the second time. But it never reassigns what they put in to the variable secret. So secret is only ever going to contain the value they typed in the very first time. If you run this in the console you'll noticed that if you type sesame the first time, you'll be asked again. But then it will quit asking. However, if you do not type in sesame the first time you'll end up in an endless loop.
I see Daryl Carr has also posted an excellent explanation. But thought it might be helpful to point out what your code is currently doing. Good luck!