Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial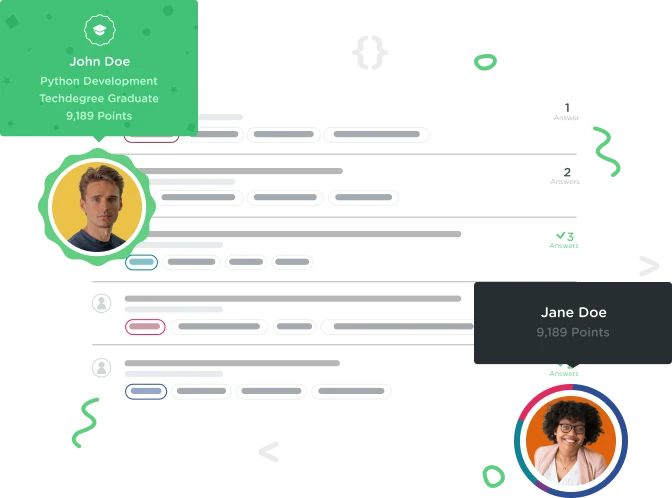
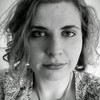
Grace Kelly
33,990 PointsHow to access object keys in for loop
I just completed one of the awesome code challenges set by Dave McFarland and I was just wondering is there a way to access the name of the key instead of manually typing it (e.g student, track points etc) whilst in a for loop??
Here's the objects within an array:
var students = [
{
name: "Grace",
track: "Front End Development",
achievements: 200,
points: 12000
},
{
name: "Kevin",
track: "Web Design",
achievements: 100,
points: 6000
}
]
and here is how I crafted the message:
var message = '';
for( var i = 0; i < students.length; i++){
student = students[i];
message += "<h2> Student: " + student.name + "</h2>";
message += "<p> Track: " + student.track + "</p>";
message += "<p> Achievements: " + student.achievements + "</p>";
message += "<p> Points: " + student.points + "</p>";
}
2 Answers
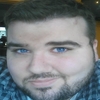
Marcus Parsons
15,719 PointsHi Grace,
There is a pretty easy way to do just that using another for loop inside of your first for loop. This second for loop is a for in loop and will iterate over the keys inside of each object and you can do exactly what you did with that data with less steps
var students = [
{
name: "Grace",
track: "Front End Development",
achievements: 200,
points: 12000
},
{
name: "Kevin",
track: "Web Design",
achievements: 100,
points: 6000
}
];
var message = '';
for( var i = 0; i < students.length; i++){
//loop through each individual object
for (var keyName in students[i]) {
//if the key name is name, add the h2 element
if (keyName === "name") {
//accesses each key within each index of the students object
//it is important that you use bracket notation here and not dot notation
message += "<h2>" + keyName + ": " + students[i][keyName] + "</h2>";
}
//otherwise give it a p element
else {
message += "<p>" + keyName + ": " + students[i][keyName] + "</p>";
}
}
}
document.write(message);
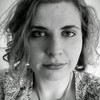
Grace Kelly
33,990 PointsOooops, sorry Marcus, when I tried out your code in workspaces it wasn't showing me the keyName, only the students[i][keyName] but i just tried it out again and it's working now, weird :s anyway sorry for the confusion and thanks a million for your help!! :)
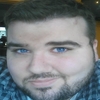
Marcus Parsons
15,719 PointsI actually modified it and used the name of the key in the code for you. That's why it didn't show before :P It's all good, Grace. I'm just glad it's working :) You're very welcome!
Geoff Parsons
11,679 PointsGeoff Parsons
11,679 PointsYou beat me to it Marcus Parsons! (Also, hello again name-brother!) Just wanted to add to your example with how to use the keys on the object:
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsHey name-brother! :D I just edited my answer as soon as you commented to adapt to her code haha
Grace Kelly
33,990 PointsGrace Kelly
33,990 PointsHi Marcus, awesome reply, thanks!! but what i was wanting to know is how to access the key names like track:, achievement: and points: instead of having to manually code them like "Track", "Achievements" and "Points" within the h2 and p tags inside the for loop...sorry if my initial question was confusing! :s I know that you can access these names in a for in loop like the following :
But trying to apply this method WITHIN a for loop is tripping me up....
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsI am severely confused by what you're asking, because in my code above, none of the names are being hardcoded at all. I am doing exactly what you asked for in my code. The code is looping through each of the keys inside each object and using those to form your message.
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsThe first for loop iterates (or goes through) the entire students array. You notice that you have multiple objects (they use { and }) and each object is separated by a comma. Data presented in this way follows the same indexing that regular arrays do. The first object is located at index 0. The second is at index 1, etc. etc. So, with that in mind, the first loop is going over each object but not inside each object.
In comes the second for loop, the "for in" loop which grabs each key from inside an object and allows you to perform commands with that data. If you notice, you are 2 layers deep into the original students array, which means that you have to use 2 sets of brackets to access the data. You have to use brackets when working with variables (such as keyName) but you can use dot notation if you reference the exact name of the key.