Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial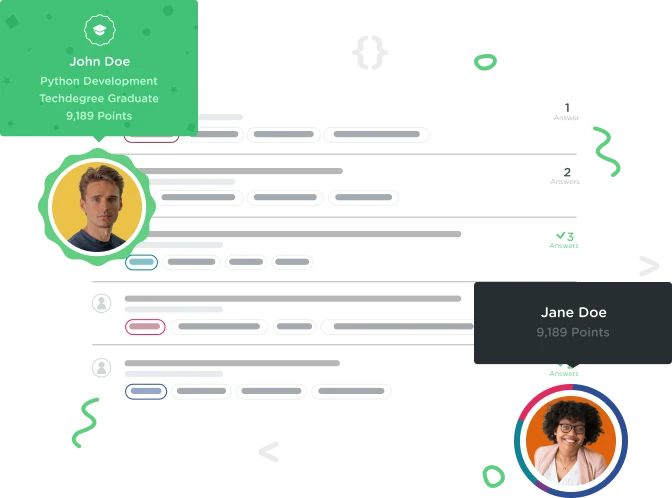
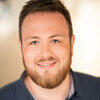
Jacob Herper
94,150 PointsHow to bind variable to the prepared statement?
I get the following error:
Bummer! Make sure you BIND the variable to your prepared statement.
My code:
<?php
require __DIR__ . '/inc/bootstrap.php';
//add function here
function deleteBook($id) {
global $db;
try{
$query = "DELETE FROM books WHERE id = '$ids'";
$stmt = $db->prepare($query);
$stmt->execute();
return $stmt->fetchAll();
} catch (\Exception $e) {
throw $e;
}
}
How can I achieve this?
<?php
require __DIR__ . '/inc/bootstrap.php';
//add function here
function deleteBook($id) {
global $db;
try{
$query = "DELETE FROM books WHERE id = '$ids'";
$stmt = $db->prepare($query);
$stmt->execute();
return $stmt->fetchAll();
} catch (\Exception $e) {
throw $e;
}
}
Ben Payne
1,464 PointsBen Payne
1,464 PointsHey Jacob,
The statement object has a
bindParam
method. If possible, you should stick with the parameter naming convention detailed in the docs PDO Docs.Also, catching the exception just to throw it again isn't doing anything. If you don't need to handle the exception just unwrap the implementation. Otherwise, you should handle the exception and log it or do something with it.
Hope that helps.
-Ben