Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial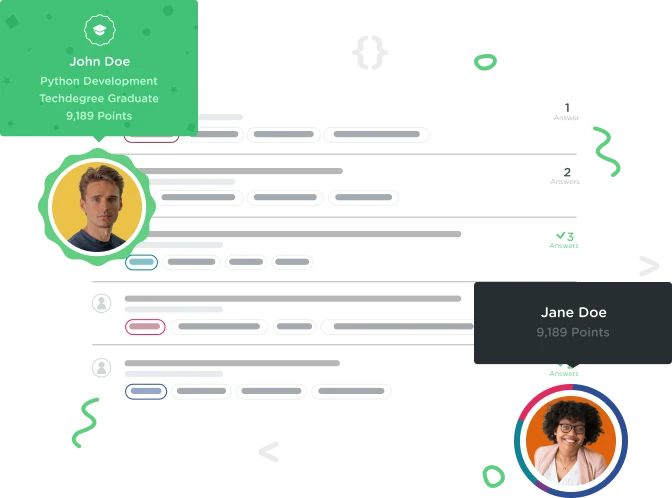

Tom Finet
7,027 PointsHow to call google endpoints from android client?
My android app uses google endpoints as a scalable backend solution. However, I am having trouble calling it from the android client.
I call an async task class from an activity and in the async task class call the endpoints method. In this case the default sayHi() method is called and the message should be displayed in a toast using the onPostExecute(String result) method.
However when I run the app module in the emulator, this error appears in the toast:
failed to connect to /10.0.2.2 (port 8080) after 20000ms: isConnected failed: ECONNREFUSED (Connection refused)
Here is the code that calls the async task from the activity:
Profile profile = new Profile(firstName, lastName, birthday); new EndpointTask(). new SaveProfileTask(profile, this).execute(); Here is the async task class:
public class EndpointTask {
public class SaveProfileTask extends AsyncTask<Void, Void, String> {
private final String LOG_TAG = SaveProfileTask.class.getSimpleName();
private MyApi mApi;
private Profile mProfile;
private Context mContext;
public SaveProfileTask(Profile profile, Context context) {
mProfile = profile;
mContext = context;
}
@Override
protected String doInBackground(Void... params) {
if (mApi == null) {
MyApi.Builder builder = new MyApi.Builder(AndroidHttp.newCompatibleTransport(),
new AndroidJsonFactory(), null)
// options for running against local devappserver
// - 10.0.2.2 is localhost's IP address in Android emulator
// - turn off compression when running against local devappserver
.setRootUrl("http://10.0.2.2:8080/_ah/api/")
.setGoogleClientRequestInitializer(new GoogleClientRequestInitializer() {
@Override
public void initialize(AbstractGoogleClientRequest<?> abstractGoogleClientRequest) throws IOException {
abstractGoogleClientRequest.setDisableGZipContent(true);
}
});
mApi = builder.build();
}
try {
return mApi.sayHi(mProfile.getFirstName()).execute().getData();
} catch (IOException e) {
return e.getMessage();
}
}
@Override
protected void onPostExecute(String result) {
Toast.makeText(mContext, result, Toast.LENGTH_LONG).show();
Log.d(LOG_TAG, "ERROR: " + result);
}
}
} Most of this is taken from a github repo, which is part of the official android documentation for hooking up the client with the backend for google app engine.
Why is this error occurring and how can it be fixed?
Thanks guys, happy coding.
1 Answer
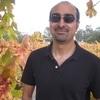
Kourosh Raeen
23,733 PointsHi Sarah - Is the backend server running? What happens when you go to localhost:8080 in a browser?
Tom Finet
7,027 PointsTom Finet
7,027 PointsYes it works fine in the browser at localhost:8080 and in API Explorer. However not with the emulator. Why? Is it something to do with permissions, not having the ANDROID_CLIENT_ID or something?
Kourosh Raeen
23,733 PointsKourosh Raeen
23,733 PointsAre you using Android Studio's built-in emulator or Genymotion?
Tom Finet
7,027 PointsTom Finet
7,027 PointsHi,
I am using android studios built in emulator.
Kourosh Raeen
23,733 PointsKourosh Raeen
23,733 PointsDo you have the following line in the manifest?
<uses-permission android:name="android.permission.INTERNET" />
Tom Finet
7,027 PointsTom Finet
7,027 PointsYes I do.
Kourosh Raeen
23,733 PointsKourosh Raeen
23,733 PointsDoes your settings.gradle file look like this, assuming you've called the Google Cloud module "backend":
include ':app', ':backend'
Also, do you have the following line in the app's build.gradle file's dependencies section, again assuming that you've called the api module "backend":
compile project(path: ':backend', configuration: 'android-endpoints')
Tom Finet
7,027 PointsTom Finet
7,027 PointsIt seems as if I fixed it somehow. I think I was missing a client id.
Kourosh Raeen
23,733 PointsKourosh Raeen
23,733 PointsGlad you figured it out :) I'm curious where the client id was missing from?