Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial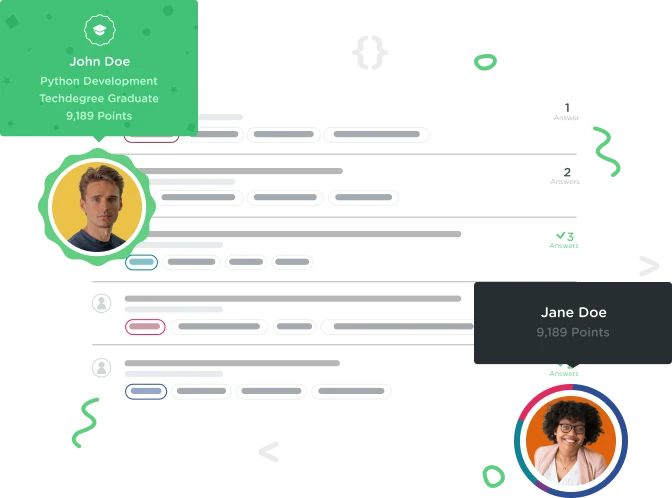

sahil jagdale
Courses Plus Student 1,043 Pointshow to check given argument is int or not ?
def squared(argument):
argument = int(input("enter any argument "))
while argument == int:
try:
answer = argument * argument
print("answer is {} ".format(answer))
except valueError :
answer = argument * len(argument)
print("answer is {} ".format(answer))

Mohamed THIAM
1,091 PointsCan someone please explain to me the difference between:
def squared(arg):
try:
if arg == int(arg):
return int(arg) ** 2
except ValueError:
return arg * len(arg)
and
def squared(arg):
try:
if int(arg):
return int(arg) ** 2
except ValueError:
return arg * len(arg)
Thanks!
6 Answers
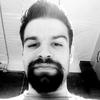
Jeffery Austin
8,128 PointsThis is what you want to do. Here, try
will multiply the argument if it can be converted to an integer. In the case that it cannot be converted to an integer you will get a ValueError, we want to tell python what to do if we get the ValueError
. So we use except ValueError:
In our case we want to multiply the string by the length of the string.
def squared(argument):
try:
answer = int(argument) * int(argument)
return answer
except ValueError:
answer = argument * len(argument)
return answer

James Gill
Courses Plus Student 34,936 PointsSahil,
Googling for "python is argument integer" will give you several good answers to your question. I say this because you're going to find that you do that often, and it often leads to Stack Overflow. And often, you'll find well thought out answers with specific examples.

Ken Alger
Treehouse TeacherSahil;
Welcome to Treehouse!
Based on your code, you are on the right track with just a few syntax issues. Let's have a look...
Task 1
This challenge is similar to an earlier one. Remember, though, I want you to practice! You'll probably want to use try
and except
on this one. You might have to not use the else
block, though.
Write a function named squared
that takes a single argument.
If the argument can be converted into an integer, convert it and return
the square of the number (num ** 2
or num * num
).
If the argument cannot be turned into an integer (maybe it's a string of non-numbers?), return the argument multiplied by its length. Look in the file for examples.
def squared(argument):
argument = int(input("enter any argument "))
while argument == int:
try:
answer = argument * argument
print("answer is {} ".format(answer))
except valueError :
answer = argument * len(argument)
print("answer is {} ".format(answer))
So your function is taking an argument, that's great. We don't need to reassign it's value though on the second line of code. while argument == int
doesn't do anything since we don't have a variable named int
, right? Further for this challenge we don't even need a while
statement.
In your try... except
statements, the challenge wants you to return an answer, not just print it out. You will also likely want to utilize the int()
method that we used previously in the course to try to convert your argument
into an integer.
Hopefully that get's you pointed in the right direction. Post back, however, if you are still stuck.
Happy coding,
Ken
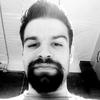
Jeffery Austin
8,128 PointsI'm thinking this is what you are trying to accomplish, If you are using input to get your number to square you won't need to use a parameter. If you run this script it will ask for a number to square. If a number isn't entered it will print that a number wasn't entered and will return to the top of the function. Once a number is entered it will print the answer.
def squared():
try:
argument = int(input("Enter a number to square "))
except NameError:
print("You didn't enter a number!")
return squared()
answer = argument * argument
print("Your answer is " + str(answer))
squared()

Ken Alger
Treehouse TeacherJeffrey, you're on a similar track, but that is not what the challenge is asking us to do. In fact, running that code in the challenge causes EOFError
s.
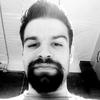
Jeffery Austin
8,128 PointsOh okay, my fault I wasn't sure what the challenge was when I posted. I was trying to go off what he was trying to do with his code.

Ken Alger
Treehouse Teacher No problem, just wanted to make sure folks aren't confused.

Barry Lam
14,406 Pointsdef squared(arg):
try:
if int(arg):
return arg * arg
except ValueError:
return arg * len(arg)
Hi Ken,
I got a TypeError: can't multiply sequence by non-int of type 'str'. Am I missing something here? Since the if statement becomes false when I put a string it, shouldn't the except function execute?
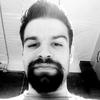
Jeffery Austin
8,128 PointsI believe if int(arg):
enters the if block if a number as a string is entered as a parameter and is trying to multiply a number as a string and a number as a string for example:
'5' * '5'
because the string '5' can be parsed into an int with int('5'), once in the if block it tries multiplying the original value, which in this example is still the string '5'
This would be what you want to do to ensure that you don't get the error.
def squared(arg):
try:
if int(arg):
return int(arg) * int(arg)
except ValueError:
return arg * len(arg)

Daniel Abildgaard
431 PointsThis also work
def squared(num):
try:
return int(num) **2
except ValueError:
return num * len(arg)
Ken Alger
Treehouse TeacherKen Alger
Treehouse TeacherEdited for markdown.