Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial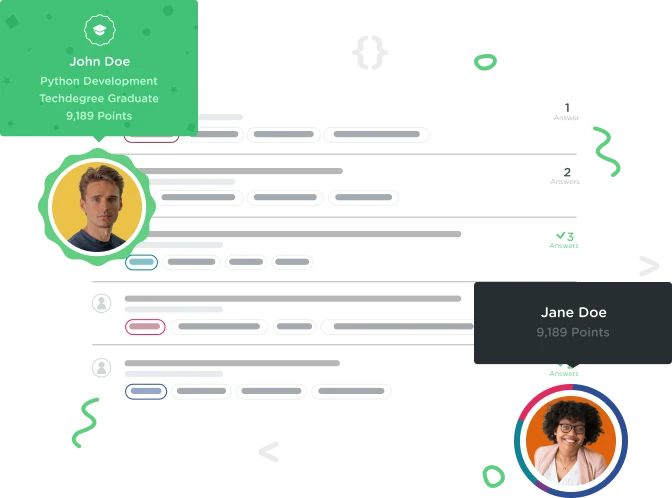

Erik Luo
3,810 PointsHow to have both string and number as argument?
How to do this?
# EXAMPLES
# squared(5) would return 25
# squared("2") would return 4
# squared("tim") would return "timtimtim"
# A function with one argument
# if is integer, convert and return the square of the number
# if is not integer count the length and multiplied by it self, then return
def squared(both):
try:
both = str or int
except ValueError:
return True
except TypeError:
return True
if both = int:
return (both * both)
elif both = str:
return (len(both) ** 2)
1 Answer
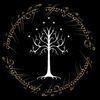
jacinator
11,936 PointsDon't overthink this.
def squared(both):
try: # The entire try/except isn't really doing much right now
both = str or int # This isn't going to do anything except assign both to str, meaning `both(3)` will return `"3"`.
except ValueError:
return True
except TypeError:
return True
if both = int: # Invalid syntax
return (both * both) # I don't think that you can multiply a class by itself
elif both = str: # Invalid syntax
return (len(both) ** 2) # Classes don't have lengths
This code will work for you.
def squared(arg):
try:
# If it's a number or can be converted to one, return the square of the number
return int(arg) ** 2
except ValueError:
# Otherwise return the argument multiplied by it's length
return arg * len(arg)
Erik Luo
3,810 PointsErik Luo
3,810 PointsIt worked, but I have some questions. First, why won't "both = str or int" work? I thought it define both as either a string or an integer. Second, why is "if both = int:" and "if both = str:" Invalid syntax? And what's the correct way to do it? Third, why you don't have to define arg? Like "arg = "? Thank you.
jacinator
11,936 Pointsjacinator
11,936 PointsWhy won't "both = str or int" work?
str
andint
are Python objects. I'm not sure if you've gotten that far yet. Saying thata = b or c
has Python look at bothb
andc
. It then finds out ifb
is Truthy andc
is Falsey or vice versa. In that case it assigns the Truthy value. If bothb
andc
are Truthy or both are Falsey it will assign the first one. This doesn't work with class objects since they are Truthy. So it entirely ignores, in your case,int
. but this only overwritesboth
as astr
object. It doesn't confirm thatboth
is a string.Why is
if both = int:
andif both = str:
invalid syntax?Correct syntax is
if both == int:
andif both == str:
. Note that the operand uses double==
and not single. This still won't do what you want, you would want to use the built in functionisinstance
, as inif isinstance(both, int):
andif isinstance(both, str):
.Why you don't have to define arg? Like
arg =
?It's because
arg
is the argument that I passed into the function. Where you usedboth
I usedarg
. Different name for the same thing.Erik Luo
3,810 PointsErik Luo
3,810 PointsThank you so much! I get it now!