Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial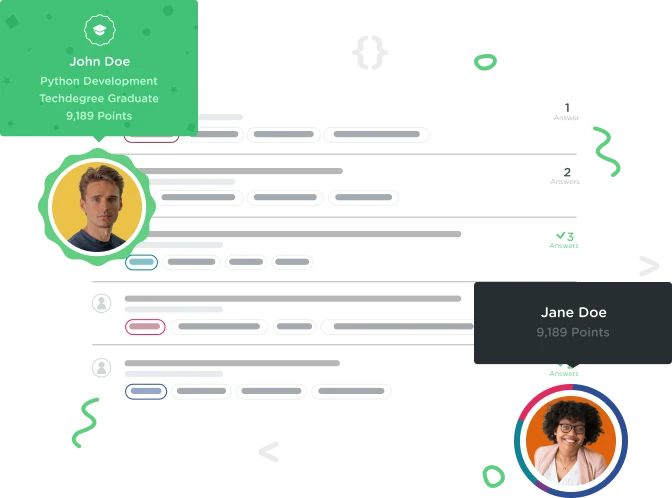

Andrew McLane
3,385 Pointshow to stop the while loop
So I added a while loop so that the loop could run for words that had multiple vowels, and multiple of the same vowels. However, I can't figure out to to get the loop to stop once all the vowels are deleted.
def disemvowel(word):
while True:
try:
word.remove("a")
word.remove("e")
word.remove("i")
word.remove("o")
word.remove("u")
word.remove("A")
word.remove("E")
word.remove("I")
word.remove("O")
word.remove("U")
except ValueError:
pass
return word
2 Answers
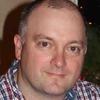
jonlunsford
16,746 PointsThe python 'break' statement is used to break out of a loop. Or you could use a boolean value outside of the loop like this.
keepLooping = True
while keepLooping:
# Loop statements here
keepLooping = False
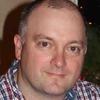
jonlunsford
16,746 PointsAndrew: The way you are going about this is awkward. For one, you are jumping into a loop instead of looping through the list. Correct me if I'm wrong but I believe the value passed in is a string. So, here is how I would solve that part.
def disemvowel(word):
# put the vowels that will be removed into a list
vowels = ['a', 'e', 'i', 'o', 'u', 'A', 'E', 'I', 'O', 'U']
# then convert the string into a list
word = list(word)
# loop through each vowel and remove it from the word
for letter in vowels:
try:
word.remove(letter)
except ValueError:
continue
# then you need to rejoin the remaining word list back into a string before you return it
return ''.join(word)
NOTE: that this code will only remove the first letter found in the word. In other words, if a word contains more than one 'a', then one will still remain. To solve this I added a second word.remove(letter) statement below the first to remove duplicate.
Andrew McLane
3,385 PointsAndrew McLane
3,385 Pointsright, I would use a break statement at the end, but how do I ensure that the loop ran enough times to delete all instances of a vowel in a word before I break?