Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial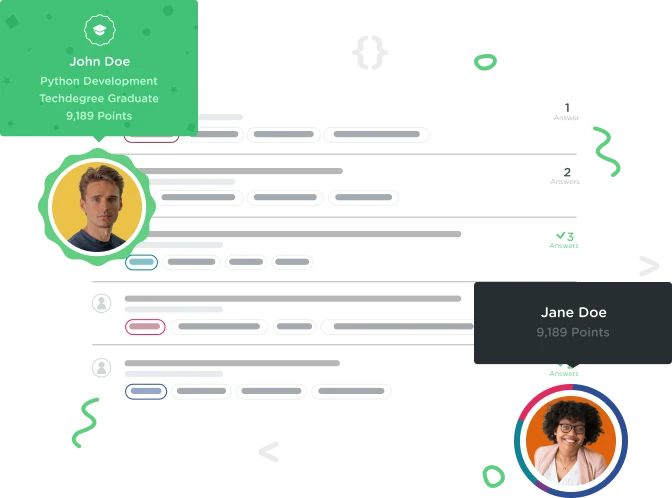

Marcos Raj
Courses Plus Student 5,979 PointsHow to upper or lower case the arrays?
var inStock = [ 'orange', 'Grape Fruit', 'Mango', 'Kiwi', 'WaterMelon', 'Banana', 'Abood', 'Sweet Melon']; var search; inStock.toUpperCase(); while(true) { search = prompt("Search for a product in our store. Type 'list' to show all the product and 'quit' to 'exit'");
if(search === 'quit')
break;
else if(search === 'list')
{
{
var listHTML= '<ul>';
for(var i = 0; i <= inStock.length;i +=1 )
{
listHTML += '<li>' + inStock[i] + '</li>';
}
listHTML += '<ul>';
document.write(listHTML);
}
break;
}
else
{
if(inStock.indexOf( search.toUpperCase()) > -1 )
{
document.write('Yes! We have the product');
}
else{
document.write('No! We Dont have the product');
}
}
break;
}
Above in the code i want to make my array to uppercase how can i do that? or I want, when i show the list items it must shows as it is, but when i search the item i want everything in uppercase? Thanks in advance.
1 Answer

Ross King
20,704 PointsHi Marcos,
You can achieve this with map(). It will leave the original array as is an only convert to uppercase in your conditional statement.
// Set variables
var inStock = [ 'orange', 'Grape Fruit', 'Mango', 'Kiwi', 'WaterMelon', 'Banana', 'Abood', 'Sweet Melon'];
var search = prompt("Search for a product in our store. Type 'list' to show all the product and 'quit' to 'exit'");
// Use map method to convert array to uppercase, then check index of search in uppercase. This does not modify the original array.
if (
inStock.map(function(item) {
return item.toUpperCase();
}).indexOf(search.toUpperCase()) > -1
) {
alert('Yes! We have the product');
} else {
alert('No! We Dont have the product');
}
see Array.prototype.map(). This may be a little confusing, if you want me to break it down a little more please let me know.
Marcos Raj
Courses Plus Student 5,979 PointsMarcos Raj
Courses Plus Student 5,979 PointsOk, so now the map is the function and inside that function we declare another function ("do we need to give the function name?"), we pass the parameter for the function, and in the body of that function whatever relevant command will give, that will directly goes to the ARRAY.
What is the purpose of passing the parameter in function "function(item)". Is it possible if don't want to pass the parameters and do the same thing?
Marcos Raj
Courses Plus Student 5,979 PointsMarcos Raj
Courses Plus Student 5,979 PointsAlso please let me know if the items are two dimensional then also we use the method as same as it is.