Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial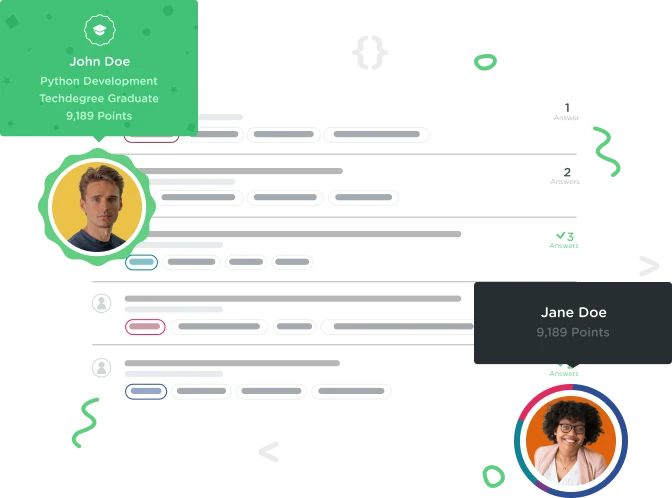

sachin bhat
Courses Plus Student 2,224 Pointshow to use float function inside the add function in the code challenge.
I am trying to add float() inside add function to convert two of its argument into floats but its giving add returned wrong values error (in code challenge)
def add(num1 , num2):
float(num1)
float(num2)
return (num1 + num2)
2 Answers
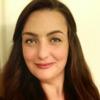
Jennifer Nordell
Treehouse TeacherHi there! You are on the right track! The problem here is that you are converting (or trying to) to float. But then you never do anything with the resulting value. There's a way we can do this that looks much like your original code:
def add(num1 , num2):
num1 = float(num1)
num2 = float(num2)
return (num1 + num2)
This will convert the number and then assign the conversion back into that variable. Then you send away the converted values. But... there's an even shorter way to do this.
def add(num1 , num2):
return float(num1 )+ float(num2)
Here we convert num1
and convert num2
and return the resulting addition all on one line.
Happy coding!

zy93
Python Web Development Techdegree Student 6,879 PointsWhen you use float(x), this returns the value as a float -- It doesnt change the value in place.
Therefore, you need to just do the following:
def add(num1, num2):
num1 = float(num1)
num2 = float(num2)
return num1 + num2
Hope this helps!