Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial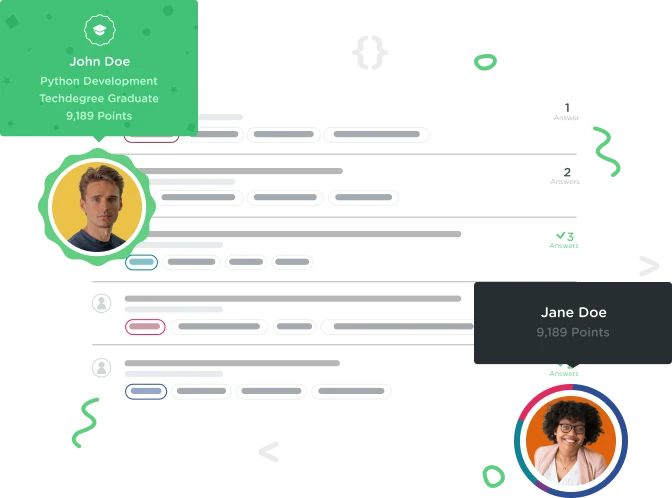
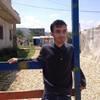
Hussein Elhussein
Courses Plus Student 2,982 Pointshow to write multidimensional array ?
i'm really stuck with this kind of arrays, maybe since more than 6 hours ago !!! so my question is if i have multidimensional array like so: $arrayName = ["value1", ["value2"]]; echo $arrayName[0][0];
i get like 1 letter, so how are the arrays and their values indexed by default?
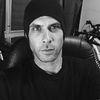
jack AM
2,618 PointsI believe the problem you're running into is the way that you're assigning the values to $arrayName. For example if you try...
$arrayName["value1"]["value2"] = "someValue";
echo $arrayName["value1"]["value2"];
You'll be able to access the value(s) according to how I think you're trying to retrieve it, as an associative array. Or...
echo $arrayName[0][0] = "value1";
echo $arrayName[0][0];
is another way that can assign values, as a numeric array :)
2 Answers
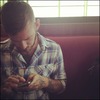
Erik McClintock
45,783 PointsHussein,
Arrays are very tricky data structures to understand, so don't feel down for having trouble with them! Even once you "understand" them, you will likely still have issues with them from time to time, particularly when we get into multidimensional arrays. You are not alone, my friend!
That said, let's poke around a bit and see if we can't get started on the path to understanding how to work with these guys.
As you know, arrays store their values at indices by default, starting at 0 (at least, in PHP, though not every language follows that same indexing methodology). So the first value in our array is stored at index 0, then index 1, then index 2, and so on. The string datatype in PHP allows you array-like access to strings in some regards, one of which is indexing. This is the reason that you're getting one letter printed out when you echo $arrayName[0][0]
.
It can be a little easier to understand if we apply some unique values to our strings. Take a look below:
$myArr = array(
'abc',
'def',
array(
'ghi',
'jkl'
)
);
echo '$myArr[0]: ' . $myArr[0] . '<br>';
echo '$myArr[1]: ' . $myArr[1] . '<br>';
echo '$myArr[2]: ' . $myArr[2] . '<br>';
echo '$myArr[3]: ' . $myArr[3] . '<br>';
Above, we created an array (called $myArr), and gave it three values. The first two values are strings, while the third is another array (thus giving us a 2D, or multidimensional, array). Can you guess what the four echo statements beneath the array will print?
. . . . .
The first statement will print out 'abc', the second will print out 'def', the third will print out 'Array' or something informing you that the item stored at that index ($myArr[2]) is an array, and the fourth statement will give you an error, since there is no fourth item in our array ($myArr[3] does not exist). Thus, we see the correlation between the indices and their values in an array: $myArr[0] prints the value stored in the very first index, $myArr[1] prints the value stored in the next index, and so forth. You are able to print, manipulate, or do whatever with the value stored at a given index when you refer to it. As mentioned, PHP gives you "array-like access" in some regards to its string datatype, with one of those points of access being indices. Thus, when you write something like $arrayName[0][0]
, you're saying: "look in the array called $arrayName. look at the value in the very first position (index 0) of that array, and then look at whatever is in the very first position of THAT (index 0 of your index 0 item of your $arrayName array)." Because the value stored at index 0 here happens to only be a string, that means you will simply get back the first character of that string.
$myArr = array(
'abc',
'def',
array(
'ghi',
'jkl'
)
);
echo '$myArr[0][0]: ' . $myArr[0][0] . '<br>';
echo '$myArr[0][1]: ' . $myArr[0][1] . '<br>';
echo '$myArr[0][2]: ' . $myArr[0][2] . '<br>';
echo '$myArr[0][3]: ' . $myArr[0][3] . '<br>';
In the above code snippet, I have revised the previous code to now look through and print out the values stored in the first, second, third, and fourth indices of the first item in our array. Can you guess what each echo statement will print?
. . . . .
The first echo statement will print 'a', the second will print 'b', the third will print 'c', and the fourth will give an error, because there isn't a fourth character (i.e. there is no fourth index) in our string.
If instead we target the third index of our base array, which happens to also be an array itself, we will get different results (which are the results you're looking for):
$myArr = array(
'abc',
'def',
array(
'ghi',
'jkl'
)
);
echo '$myArr[2]: ' . $myArr[2] . '<br>';
echo '$myArr[2][0]: ' . $myArr[2][0] . '<br>';
echo '$myArr[2][1]: ' . $myArr[2][1] . '<br>';
echo '$myArr[2][2]: ' . $myArr[2][2] . '<br>';
Can you guess what these will echo? Remember, each index is going to put you into the given value, then look to the appropriate item at the next given index...
. . . . .
Our first echo statement will again print out "Array" or a notice informing us that that is what is stored at that index. The second echo statement, though, will print out 'ghi', the third statement will print out 'jkl', and the fourth will again print an error, as there is no third item in this array. Do you see how that works? It looks and feels a little weird, and it can be tricky to get used to, but if you break it down, you'll notice that it is exactly the same as printing a full value from the base array, with the one caveat that you have to target the array with its index as well before you get a value from it!
So let's say we have the following array:
$employees = array(
array(
'andrew',
'javascript'
),
array(
'nick',
'html'
),
array(
'guil',
'css'
)
);
How would you print each name and language to the screen? Notice that there are no raw values in the base array; you will have to access each value first by its array's index, then its own index. See if you can get each of those values, and let me know!
Hope this has helped some, and happy coding!
Erik
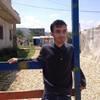
Hussein Elhussein
Courses Plus Student 2,982 Pointsthanks Erick so much, helped me alot, and this is how i get them:
echo $employees[0][0];
echo $employees[0][1];
echo $employees[1][0];
echo $employees[1][1];
echo $employees[2][0];
echo $employees[2][1];
i think my problem was, trying to point to the base array index, which it doesn't have one
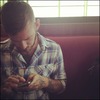
Erik McClintock
45,783 PointsHussein,
Those echo statements are indeed correct! Hopefully things will be a bit more clear for you regarding arrays from here on, but remember: If you come across a problem or something confusing with them again, you're not the only one!
Happy coding!
Erik
Nelson Lee
6,853 PointsNelson Lee
6,853 PointsSince your using php
This is how you write a 2 dimension array.
$myArray = array( array(items,...), array(items,...), array(items,...) );
// The bottom code explains it in javascript
The array you and the part your echoing is correct. Here is what is happening.
$arrayName[0] is "value1" (returns the string) $arrayName[1] is ["value2"] (returns the subarray)
if you did $arrayName[0][0] your applying the index towards the string value.
"value1"[0] which is equal to "v"
if you wanted it to return the value you need to add brackets like this
$arrayName = [["value1"], ["value2"]];
$arrayName[0] is ["value1"]; $arrayName[0][0] is "value1";
Assume you had $arrayName = [["value1", "value2"], ["value2"]];
To access value2 you need $arrayName[0][1] is "value2"