Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial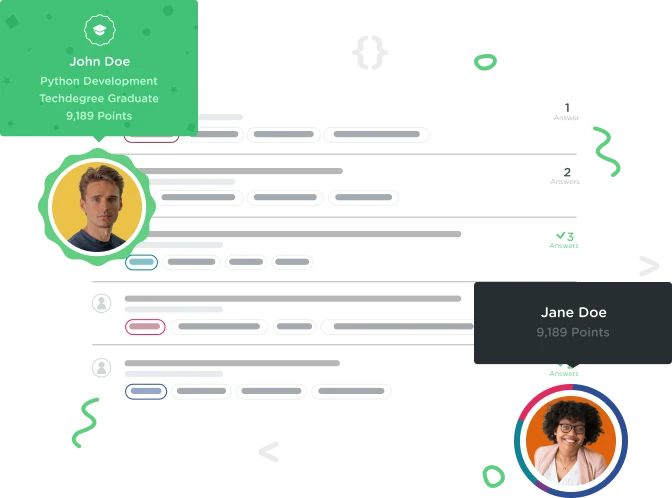

Dominick McCleary
3,012 Pointshow would i do this square function
i guess its kinda bother some to do this but i really am trying to figure out how to do this and i spent a while trying to figure it out how would i properly type this function cause every time i try to type it i come up with this
# EXAMPLES
# squared(5) would return 25
# squared("2") would return 4
# squared("tim") would return "timtimtim"
def squared(num):
try:
num = int(input("Give a number "))
if squared.int == True:
return (num ** num)
except ValueError:
return (str(num) ** str(num))

Dominick McCleary
3,012 Pointshow would i do the second part exactly

Benjamin Lange
16,178 PointsThe function that you need to use is the len() function. This function can be used on lists and strings and other objects to return the length of that object. For example, len("Dominick") would return 8 and len([1, 2, 3]) would return 3.

Dominick McCleary
3,012 Pointsso i would have to put the variable num into a string before squaring it

Benjamin Lange
16,178 PointsNo, you won't have to. In this exercise, the variable num can be passed to the square function as either an int or a string. If it is an int, the code in the try block will run. If it is not an int (so num is a string), the code in the except block will run.
In a previous lesson, we learned that you can multiply a string by a number to repeat the string that many times. So, "Ben" * 3 would return BenBenBen. You can't multiply a string by a string, but you can use the len() function to get the length of the string num and then multiply that by the length of the string num.
Is that making a little more sense?

Dominick McCleary
3,012 Pointskinda so it would look like len(num) * num

Benjamin Lange
16,178 PointsExactly.

Dominick McCleary
3,012 Pointsi have done that multiple times and it doesnt accept it at all

Benjamin Lange
16,178 PointsPost your most updated code snippet so that we can take a look.

Dominick McCleary
3,012 Pointshttps://teamtreehouse.com/library/python-basics/number-game-app/squared and i got it to where squared is no longer returning the right answer

Benjamin Lange
16,178 PointsOK. You'll have to post your actual code here though. That link brings up my solution for that exercise, not yours.

Dominick McCleary
3,012 Pointsdef squared(num):
try:
num = int("Give me a number ")
return num * num
except ValueError:
x = str(num)
return len(x) * num
squared('num')

Benjamin Lange
16,178 PointsOK, three things:
- You don't need to convert the variable num into a string in the except block. If it gets to the except block, num will be a string. You don't need that line.
- The return in the except isn't quite right. You just want to return len(num) * num
- You do not need to prompt the user for input. The num variable is passed into the function by Treehouse.
I recommend that you get this working and then go back and review some of the previous videos and exercises to solidify your understanding.

Dominick McCleary
3,012 Pointsok im getting everything done how you say but it keeps saying that squared is not returning the right answer

Benjamin Lange
16,178 PointsPost your updated code again. It also might be helpful to you to use a Treehouse Workspace to write the code for this. You can save your code into a file and then run the code to test it out.

Dominick McCleary
3,012 Pointsdef squared(x): try: return x * x except TypeError: return len(x) * x

Benjamin Lange
16,178 PointsYou're very close!! In the try block, use int(x) to try to convert the argument into an int. It looks like if Treehouse passes in a string "2" that it should try to convert it into a number. Also, you want to catch a ValueError instead of a TypeError.

Dominick McCleary
3,012 Points[def squared(x): try: return int(x) * x except ValueError: return len(x) * x] like this?

Benjamin Lange
16,178 PointsIn the try block, change the second x to be int(x) also, and you should be good to go!

Dominick McCleary
3,012 Pointsthank you!

Benjamin Lange
16,178 PointsYou're welcome! I know that learning to program can get frustrating and there's so much to learn, but it is so rewarding when you work on a project and put together something new. Keep up the hard work!!
Benjamin Lange
16,178 PointsBenjamin Lange
16,178 PointsIn the try block, you are already attempting to convert the input to an int. The if statement below that line is unnecessary. simply remove that line. If the conversion to int fails, it will raise the ValueError exception and it will move on to the except block.
Down in the except block...You cannot square a string object, it will return an unsupported operand error. What you want to do instead is determine the length of the string and return the string times that number. (num * length of num) I hope this helps!