Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial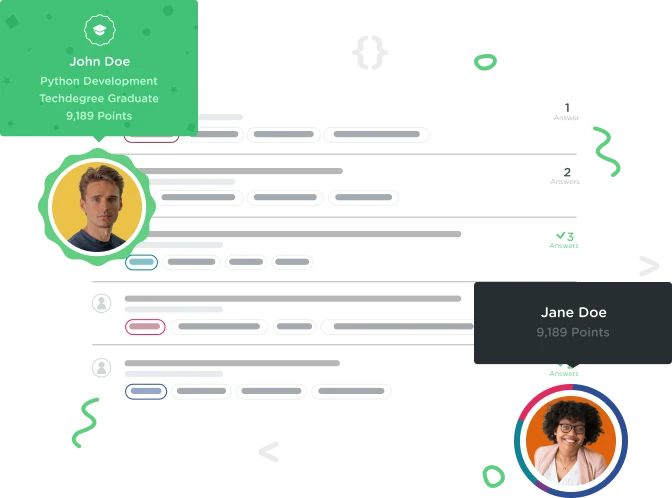

Maria Matongo
8,380 Pointshttps://teamtreehouse.com/library/javascript-basics/making-decisions-with-conditional-statements/super-conditional-chall
please help
var money = 9;
var today = 'Friday'
if ( money >= 100 || today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 || today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 || today === 'Friday' ) {
alert("Time for a movie");
} else if ( money < 9 || today=== 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
1 Answer

andren
28,558 PointsThere are two issues with the code the challenge presents you with, you have fixed one of the issues (though you have also introduced another issue) but the other issue remains.
All of the if statements use the OR (||) operator, the way that operator works is that it will return true, and therefore run the if statement, if either of the conditions supplied are true. Since the first if statement checks if the day is Friday (which it is) it will always run, regardless of how much money there is because of the OR operator.
To fix that issue you have to change the OR (||) operator to an AND (&&) operator. The AND operator does the opposite of the OR operator. It will only return true if both of the conditions supplied are true, if either of them are false it will return false.
So the first step to solving this challenge is to change all of the || operators to &&.
The second issue present in the original code is that the last else if statement checks if it is not Friday instead of checking if it is Friday. You have fixed that issue but you have also added a new condition to the else if statement which you were not meant to do.
Fixing those two issues (without adding any new conditions) like this:
var money = 9;
var today = 'Friday'
if ( money >= 100 && today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 && today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 && today === 'Friday' ) {
alert("Time for a movie");
} else if ( today === 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
Will allow you to pass the challenge.