Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial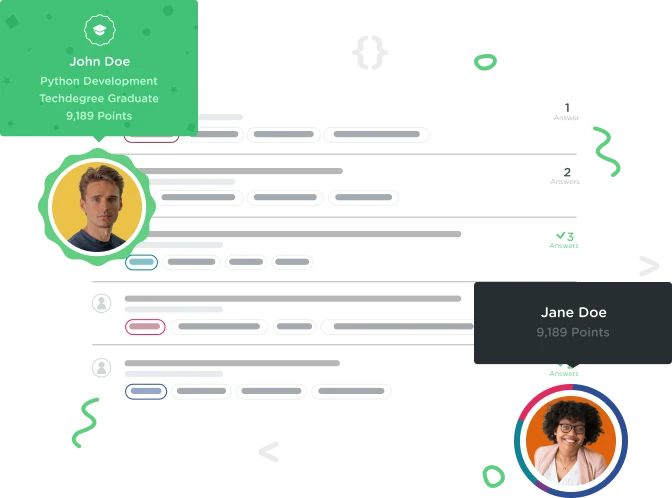
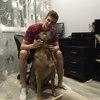
Arthur Kharzamanov
5,414 PointsI am confused about the id we create in RelativeLayout
Hello,
So I tried to change the background following the steps described in the video and I realized that I already had an id for the RelativeLayout in the xml file (android:id="@+id/activity_fun_facts"). It is even used in FunFactsActivity.java in onCreate method: (setContentView(R.layout.activity_fun_facts);).
My first question is why doesn't Ben have this id declared in xml file since it is also referenced in his onCreate method?
Second question is why since I had it declared in xml the software didn't recommend it in the code autocompletion when initializing the mRelativeLayout in the onCreate method? Once I used this id the color changed just fine. However, if I add another id in the xml under RelativeLayout it gives an error for name duplication of ids since I also say (android:id=...).
Thank you!
Here is my code:
public class FunFactsActivity extends AppCompatActivity {
private FactBook mFactBook = new FactBook();
private TextView mFactTextView;
private Button mShowFactButton;
private RelativeLayout mRelativeLayout;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts);
mFactTextView = (TextView) findViewById(R.id.factTextView);
mShowFactButton = (Button) findViewById(R.id.showFactButton);
mRelativeLayout = (RelativeLayout) findViewById(R.id.activity_fun_facts);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View view) {
String fact = mFactBook.getFact();
mFactTextView.setText(fact);
mRelativeLayout.setBackgroundColor(Color.RED);
}
};
mShowFactButton.setOnClickListener(listener);
}
}
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/activity_fun_facts"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.example.akboss.funfacts.FunFactsActivity"
android:background="#51b46d">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Did you know?"
android:textSize="24sp"
android:textColor="#80ffffff" />
<Button
android:text="Show another fun fact"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:layout_centerHorizontal="true"
android:id="@+id/showFactButton"
android:background="@android:color/white" />
<TextView
android:text="Ants stretch when they wake up in the morning"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/factTextView"
android:textSize="24sp"
android:layout_centerVertical="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:textColor="@android:color/white" />
</RelativeLayout>
1 Answer
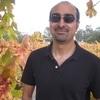
Kourosh Raeen
23,733 PointsHi Arthur - In the following line of code:
setContentView(R.layout.activity_fun_facts);
R.layout.activity_fun_facts
is not the id of the RelativeLayout. It's the name of a layout file. Notice that the name of the file is preceded by R.layout
as opposed to R.id
as in:
mFactTextView = (TextView) findViewById(R.id.factTextView);
Also, the id of the RelativeLayout is supposed to be relativeLayout
:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:id="@+id/relativeLayout"
tools:context="com.teamtreehouse.funfacts.FunFactsActivity"
android:background="#51b46d">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Did you know?"
android:textSize="24sp"
android:textColor="#80ffffff"/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Ants stretch when they wake up in the morning."
android:id="@+id/factTextView"
android:layout_centerVertical="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:textSize="24sp"
android:textColor="@android:color/white"/>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Show Another Fun Fact"
android:id="@+id/showFactButton"
android:layout_alignParentBottom="true"
android:layout_centerHorizontal="true"
android:background="@android:color/white"/>
</RelativeLayout>
Arthur Kharzamanov
5,414 PointsArthur Kharzamanov
5,414 PointsOh thats right, now I see the difference. Thank you! I got confused because I did have it set as the id in the xml file (android:id="@+id/activity_fun_facts") I didn't create it manually, so maybe I clicked something by accident.