Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial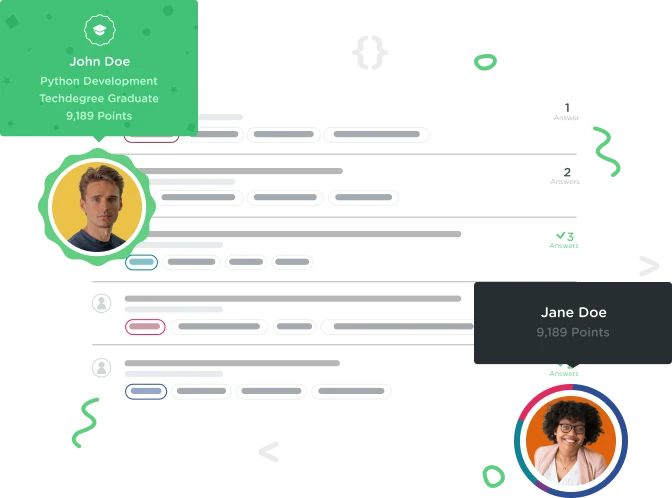

Shawn Boyd
6,907 PointsI am not getting the 4/4 on slices. Please help!!!
I am not getting the last step in the slice functions. I am not understanding what I am doing wrong.
def first_4(slice):
return slice[0:4]
def first_and_last_4(x):
return x[0:4] + x[-4:]
def odds(index):
return index[1::2]
reverse_evens(index):
rev = index[::2]
return rev.reverse[::-2]
2 Answers
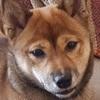
Katie Wood
19,141 PointsHi there,
A couple things here. It looks like you have the right idea, but are implementing parts of two different ways to solve this. I've tried both and they both work, so I'll go through each one:
First, there's the method you're closer to here, where you first find the evens and then just use the list .reverse method on them. For that to work, you don't need to use a negative increment - just call reverse() on your rev variable, like this:
def reverse_evens(index): #also be sure to add the 'def' keyword on this line
rev = index[::2]
rev.reverse()
return rev
The above should work - a key thing to note is that .reverse() will just reverse the list and store it in the original variable - it will not return the reversed list, so doing return rev.reverse() will return None, rather than the list. The other method you recognized in your solution was using a negative increment - this way works too, but your starting point will differ based on whether there are an even or odd number of items in the list. This can be handled with a conditional:
def reverse_evens(index):
if len(index) % 2: #if there are an odd number of items
return index[-1::-2]
else: #if there are an even number of items
return index[-2::-2]
Remember that we can define a negative index as the starting point, counting from the end of the list at index -1. If there are, say, five items in the array, then the last item will be at index 4, an even - we want to return that, so we would start with the last item, at -1. If there are six items, the last item will be at index 5, so we want to start with the one before that, at index -2. That's the general idea behind this solution.
Hopefully this helps - feel free to use whichever one you like!

Shawn Boyd
6,907 PointsIt worked!!! Thank you!!!!!!!!!