Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial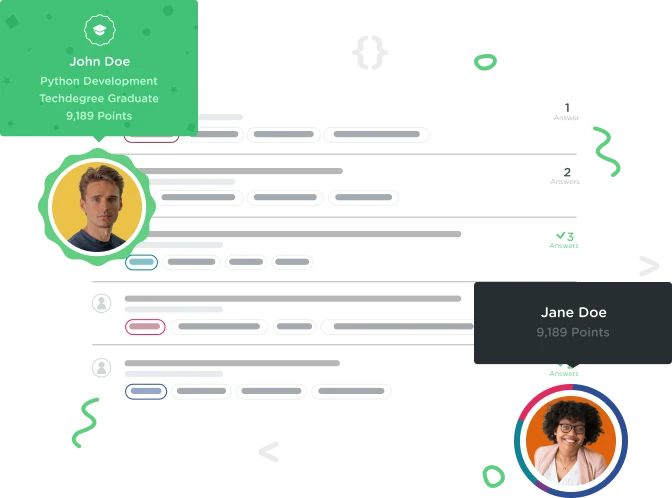
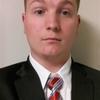
Steven Porter
3,097 PointsI can't find out what is wrong with my code!
// Student Data
var students = [
{
name: 'Frank',
track: 'JavaScript',
achievements: 277,
points: 12345
},
{
name: 'Jeff',
track: 'IOS',
achievements: 364,
points: 467456
},
{
name: 'Lucy',
track: 'PHP',
achievements: 666,
points: 666666
},
{
name: 'Steve',
track: 'Ruby',
achievements: 1,
points: 2
}
];
// Print Student Report
var message = '';
var student;
var search;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
//Search Through Student's Data
function getStudentReport(student) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
report += '<p>Points: ' + student.points + '</p>';
return report;
}
while(true){
search = prompt("Search Student's Records: Enter a name, or Enter 'exit' to exit.");
if (search === null || search.toLowercase() === 'quit') {
break;
}else {
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if(student.name === search){
message = getStudentReport(student);
print(message);
}
}
}
}
Any thoughts?
4 Answers
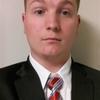
Steven Porter
3,097 PointsGotcha! Thanks for the help!
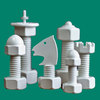
Steven Parker
241,970 PointsIt looks like you just have a typo.
Instead of "toLowerCase", you wrote "toLowercase" (with small "c").
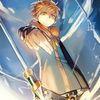
Michael Cacciano
7,349 PointsI'm a noob myself but along with what Steven Parker said when you drop this in the JS console after fixing the toLowerCase() issue if you search for a name in all lower case it doesn't return the studentReport for me. I added to the if statement at the end so all names can be searched in all caps or all lowercase.
if(student.name.toLowerCase() === search.toLowerCase())
I'm not sure if this is necessary or redundant but it works in the console in caps or lowercase :D P.S. You wrote type 'exit' to exit in the prompt but in the statement you wrote
if (search.toLowerCase() === 'quit')
as a heads up :D
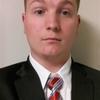
Steven Porter
3,097 PointsAfter implementing both of your contributions, I've noticed that, for me, the data is not displayed onto the screen until I either type 'quit', or click 'cancel'.
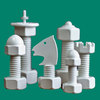
Steven Parker
241,970 PointsThat is normal behavior for modern browsers. Older versions (like when the video was mode) rendered the page while the JavaScript was running, but newer ones only render after the JavaScript finishes.