Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial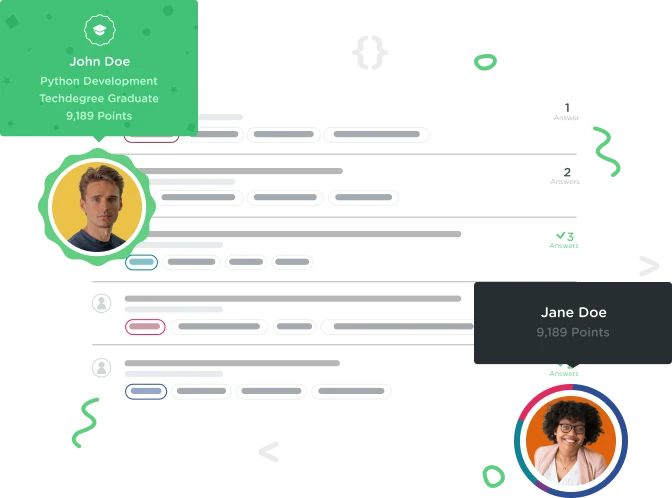

Joseph Burgan
1,312 PointsI can't get anything lower than a Silver.
If I try to get a 0, 1, or 2, it's supposed to say I get a Bronze Medal or I didn't pass, but instead it still says I have a Silver Medal. Can someone spot the problem?
// 5 questions
var answer1 = prompt("In Toy Story, what is the name of the super cool pizza place?"); // Pizza Planet
var answer2 = prompt("Who is the dude in green in The Legend of Zelda?"); // Link
var answer3 = prompt("What is GLaDOS' favourite vegetable?"); // Potatoes
var answer4 = prompt("Who is Luke Skywalker's father?"); // Darth Vader
var answer5 = prompt("Who says, 'YOU! SHALL NOT! PASS!'"); // Gandalf
var numberCorrect = 0;
// Question 1
if (answer1.toUpperCase() === "PIZZA PLANET")
{
numberCorrect += 1;
}
else{}
// Question 2
if (answer2.toUpperCase() === "LINK")
{
numberCorrect += 1;
}
else{}
// Question 3
if (answer3.toUpperCase() === "POTATOES")
{
numberCorrect += 1;
}
else{}
//Question 4
if (answer4.toUpperCase() === "DARTH VADER")
{
numberCorrect += 1;
}
else{}
//Question 5
if (answer5.toUpperCase() === "GANDALF")
{
numberCorrect += 1;
}
else{}
// Awards
if ( numberCorrect === 5)
{
document.write("Congratulations! You got " + numberCorrect + " correct out of 5 and earned a Gold Medal!");
}
else if ( numberCorrect === 3 || 4)
{
document.write("Congratulations! You got " + numberCorrect + " correct out of 5 and earned a Silver Medal!");
}
else if ( numberCorrect === 1 || 2)
{
document.write("Congratulations! You got " + numberCorrect + " correct out of 5 and earned a Bronze Medal!");
}
else if ( numberCorrect === 0)
{
document.write("Sorry, but you shall not pass. :(");
}
1 Answer
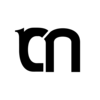
Colin Marshall
32,861 PointsIt is probably a problem with your comparisons. Here is what you have:
else if ( numberCorrect === 3 || 4)
It first checks to see if numberCorrect is equal to 3. You are missing the "numberCorrect ===" on the other side of the OR so there is no comparison being made and it is evaluating to true. That is why you have 0 correct and you are getting a silver medal.
It evaluates both sides of the || (OR) like they are enclosed in a set of parentheses:
( (numberCorrect === 3) || (4) )
You want to do this instead:
else if ( numberCorrect === 3 || numberCorrect === 4)
Joseph Burgan
1,312 PointsJoseph Burgan
1,312 PointsBIG thank you, Colin. Works perfectly! Thank you for explaining it all as well, I don't think I'll make this mistake ever again!
James Gill
Courses Plus Student 34,936 PointsJames Gill
Courses Plus Student 34,936 PointsYep, what Colin said. It's an easy mistake to make.