Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial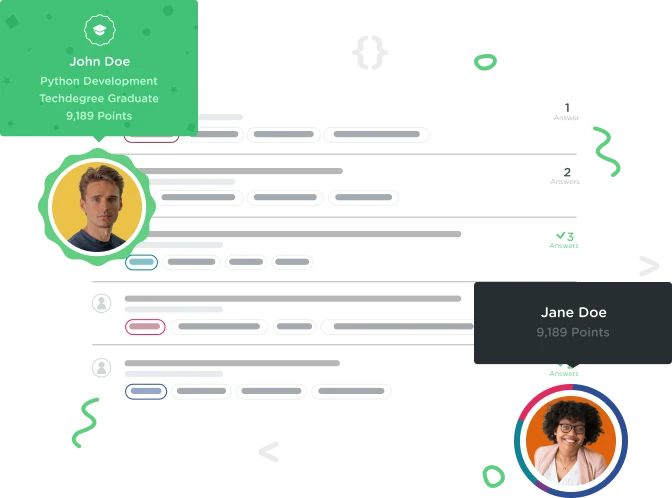
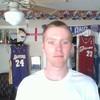
michael randell
4,119 PointsI can't get my add task button working/tweaked. Stuck.
It does add the button but it's the tweaking that I am having a problem with...I can't seem to make it all formatted properly.
Can someone please help me...Thank you guys.
//Problem: User interaction doesn't provide desired results.
//Solution: Add interactivity so the user can manage daily tasks.
var taskInput; //new-task
var addButton = document.getElementsByTagName('button')[0]; //first button
var incompleteTaskHolder = document.getElementById('incomplete-tasks'); //incomplete-tasks
var completedTaskHolder = document.getElementById('completed-tasks'); //completed-Tasks
//New Task List Item
var createNewTaskElement = function(taskString) {
//Create List Item
var listItem = document.createElement('li');
//input (checkbox)
var checkBox = document.createElement('input'); //checkbox
//label
var label = document.createElement('label');
//input (text)
var editInput = document.createElement('input'); //text
//button.edit
var editButton = document.createElement('button');
//button.delete
var deleteButton = document.createElement('button');
//Each element needs modifying
//Each element needs appending\
listItem.appendChild(checkBox);
listItem.appendChild(label);
listItem.appendChild(editInput);
listItem.appendChild(editButton);
listItem.appendChild(deleteButton);
return listItem;
}
//Add a new task
var addTask = function() {
taskInput = '';
console.log('Add task...');
//Create a new list item with the text from #new-task
var listItem = createNewTaskElement(taskInput.value);
//Append listItem to incompleteTasksHolder
incompleteTaskHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
}
//Edit an existing task
var editTask = function() {
console.log('Edit task...');
var listItem = this.parentNode;
var editInput = listItem.querySelector('input[type=text]');
var label = listItem.querySelector('label');
var containsClass = listItem.classList.contains('editMode');
//If the class of the parent is .editMode
if(containsClass) {
//Switch from .editMode
//label text become the input's value
label.innerText = editInput.value;
} else {
//Switch to .editMode
//input value becomes the label's text
editInput.value = label.innerText;
}
//Toggle .editMode on the list item
listItem.classList.toggle('editMode');
}
//Delete an existing task
var deleteTask = function() {
console.log('Delete task...');
var listItem = this.parentNode;
var ul = listItem.parentNode;
//Remove the parent list item from the ul
ul.removeChild(listItem);
}
//Mark a task as complete
var taskCompleted = function() {
console.log('Task complete...');
//Append the task list item to the #completed-tasks
var listItem = this.parentNode;
completedTaskHolder.appendChild(listItem);
bindTaskEvents(listItem, taskIncomplete);
}
//Mark a task as incomplete
var taskIncomplete = function() {
console.log('Task incomplete..');
//Append the task list item to the #incomplete-tasks
var listItem = this.parentNode;
incompleteTaskHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
}
var bindTaskEvents = function(taskListItem, checkBoxEventHandler) {
console.log('Bind list item events');
//select taskListItem's children
var checkBox = taskListItem.querySelector('input[type=checkbox]');
var editButton = taskListItem.querySelector('button.edit');
var deleteButton = taskListItem.querySelector('button.delete');
//bind editTask to edit button
editButton.onclick = editTask;
//bind deleteTask to the delete button
deleteButton.onclick = deleteTask;
//bind taskCompleted to checkbox
checkBox.onchange = checkBoxEventHandler;
}
//Set the click handler to the addTask function
addButton.onclick = addTask;
addButton.onclick
//cycle over incompleteTaskHolder ul list items
for(var i = 0; i < incompleteTaskHolder.children.length; i++) {
//bind events to list item's children (taskCompleted)
bindTaskEvents(incompleteTaskHolder.children[i], taskCompleted);
}
//cycle over completedTaskHolder ul list items
for(var i = 0; i < completedTaskHolder.children.length; i++) {
//bind events to list item's children (taskiIncomplete)
bindTaskEvents(completedTaskHolder.children[i], taskIncomplete);
}
It does add the button but it's the tweaking that I am having a problem with...I can't seem to make it all formatted properly.
Can someone please help me...Thank you guys!
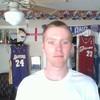
michael randell
4,119 PointsHey Michael, it is not letting my reply to your post but yea! It's the tweaking that I'm having a problem with.
1 Answer
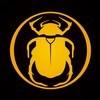
rydavim
18,814 PointsYou have a comment in your createNewTaskElement function, but haven't completed that step.
//Each element needs modifying
Is this the part you need help with? You'll need to modify each element to include things like types, text values, and element classes. Consider using element.setAttribute(name, value) to ensure your newly created elements have all their attributes setup correctly.
This should solve your issue. If you haven't gotten to this point in the lessons, you can safely continue until he covers this. Please let me know if you have any questions, or if you'd like further help. Happy coding! :)
Michal Weizman
14,050 PointsMichal Weizman
14,050 PointsHi Michael, Can you be more specific? Where are you exactly in the challange, and what does the button refuse to do? I'm asking because I just tried your code in my console, and I can see a new task created after pressing the button [It still needs some tweaking - but a new task was created when I tried it].
Try to be more specific so I'll know what to look for. I hope this helped...