Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial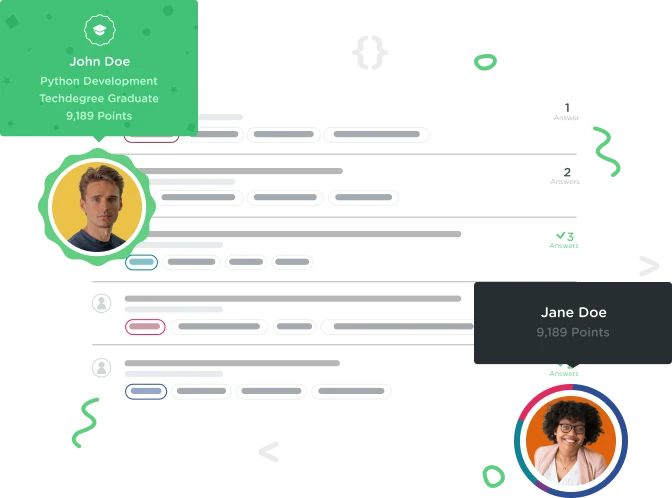

Jack Beauchamp
2,411 PointsI can't pass method Returns: Part 2 can someone give me the answer
I really want to pass this because I have already completed all the ruby basics please help
def mod(a, b, c)
#write your code here
puts "The remainder of #{a} divided by #{b} is #{c}"
return a % b = c
end
puts mod(4, 3)
puts mod{1}
11 Answers
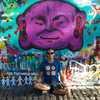
Andrew Stelmach
12,583 PointsOk, the first important thing to make a note of is that puts
returns nil
.
puts "a string"
will first print the string, but will then return nil
. Open irb in your terminal and write puts "a string"
and you will see it print "a string" and then return nil (indicated by => nil
).
Now, the challenge asked you to write a method that RETURNS the sentence with the interpolated variables. The important thing to remember is that a method returns its last evaluated statement. The last evaluated statement in your method is return a % b = c
. So, I expect your method will return whatever value is assigned to c
.
- You don't need to call the method after you've written it; you just need to write the method. Treehouse will call and test your method in the background when you submit your answer.
Have another go. Remember you can write ruby files and run them in your terminal. You can also use irb in your terminal - both important habits to get into.
Even better, learn how to use RSpec to write tests :-)
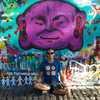
Andrew Stelmach
12,583 PointsTry this for a taste of TDD:
Open your terminal and do:
gem install rspec
mkdir mod
cd mod
rspec --init
touch mod.rb
touch ./spec/mod_spec.rb
Open the whole folder in your text editor.
Open the file .rspec
and add an extra line saying --format documentation
and save the file. This makes rspec print out passing tests to the screen.
Write your test:
#spec/mod_spec.rb
require 'spec_helper'
require_relative '../mod'
describe '#mod' do
it "returns a sentence describing the result of dividing its two arguments" do
expect(mod(5, 3)).to eq "The remainder of 5 divided by 3 is 2."
end
end
In your terminal, run your test with the command, rspec
, and watch it fail. It will say something like mod
is an undefined method.
Always follow the errors from your failing test to write your code.
Take the error message's advice and define a method called mod
:
#./mod.rb
def mod
end
Run your test again; you will get an ArgumentError exception saying there should be two arguments. So, give it two arguments!:
#./mod.rb
def mod(a, b)
end
Run your test again; you will get a really useful message this time: "expected: 'The remainder of 5 divided by 3 is 2.' got: nil"
Great! So now you can write your method. Run your test every time you think you've written your method correctly, to test your method. When you've written your method correctly you will get a beautiful green passing test and it's time to celebrate!

Jack Beauchamp
2,411 PointsThanks very helpful

James Tierney
6,940 PointsHi All, I submitted the following method for the answer to the ""Challenge. It works perfect in irb and a ruby file load into irb but fails the "Challenge". All direction will be most helpful. I tried to complete the install of "rspec" in my workspace console but it would not complete in full.
def mod(a,b) #write your code here c = a % b puts "The remainder of #{a} divided by #{b} is #{c}." return c end
kind regards,
James
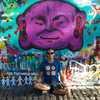
Andrew Stelmach
12,583 PointsAh are you on Windows, James?
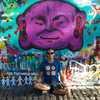
Andrew Stelmach
12,583 PointsA couple of hints - remember that a method returns its last evaluation. You're returning the value of c
. To quote the challenge: "it would be nicer if method returned the remainder in a nice full sentence. " and, "Use string interpolation to return the remainder inside the sentence “The remainder of a divided by b is c.” where a is your “a” variable, b is your “b” variable, and c is the value of a % b."
They want your method to return the sentence.
Let me know if this doesn't make it click into place in your mind.

James Tierney
6,940 PointsOsx El Capitan 10.11.4
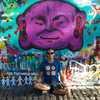
Andrew Stelmach
12,583 PointsOh, well try to get a dev environment setup on your MAC sometime - that would be awesome. You'll need to install homebrew and rvm. Let me find a good link for you to do that - you'll have to do it soon if you're serious about developing.
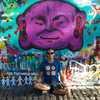
Andrew Stelmach
12,583 PointsThis looks good for getting your dev environment setup - it uses rbenv instead of rvm but that's 100% fine. Give it a shot and just follow it to the letter: https://gorails.com/setup/osx/10.11-el-capitan
Expect to hit some hurdles, but that's all part of being a developer!
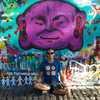
Andrew Stelmach
12,583 PointsIn fact, a really awesome task for you would be to get your dev environment setup as per that post and then do that TDD with RSpec task I laid out above. If you get all that working then you really do have all the tools ready to do just about anything for the forseeable future!
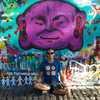
Andrew Stelmach
12,583 PointsFeel free to message me on facebook if you need help (you can find that on my Treehouse profile).

James Tierney
6,940 PointsI used homebrew to install the latest ruby and will get rails set up at a later date. I will set up rspec for troubleshooting and info on methods.
I tried the following and still no joy,
def mod(a,b)
c = a % b
ans = puts "The remainder of #{a} divided by #{b} is #{c}."
return ans
end
yet again it works fine in irb and a ruby file.
Thanks.

James Tierney
6,940 PointsOk I got it. Just a matter of naming it correctly.
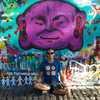
Andrew Stelmach
12,583 PointsHmm what do you mean by 'naming it correctly'? Can you post your final code? PS use Markdown to format your code when you post here.

James Tierney
6,940 PointsHello code examples are,
This code did not work for the challenge
def mod(a,b)
c = a % b
ans = puts "The remainder of #{a} divided by #{b} is #{c}."
return ans
end
This code did work for the challenge
def mod(a,b)
c = a % b
remainder = puts "The remainder of #{a} divided by #{b} is #{c}."
return remainder
end
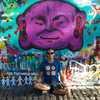
Andrew Stelmach
12,583 PointsHi James, that code definitely won't work for the challenge, because of the puts
.
Re-read my first answer where I talked about puts
- it will probably make more sense now.
Taking your above method (the second one), lets say you called that method passing in 5 and 3 as the arguments, what will happen is that "The remainder of 5 divided by 3 is 2."
will be printed to the screen and then nil
will be returned; that method will always RETURN nil. It will therefore fail the challenge.
To make that method pass the challenge, you need to make it RETURN the sentence, so:
def mod(a, b)
c = a % b
remainder = "The remainder of #{a} divided by #{b} is #{c}."
return remainder
end
This method can be made more concise; first of all, because return
is on the last line of the method, return
is not needed:
def mod(a, b)
c = a % b
remainder = "The remainder of #{a} divided by #{b} is #{c}."
remainder
end
This is even more concise:
def mod(a, b)
c = a % b
"The remainder of #{a} divided by #{b} is #{c}."
end
And, arguably, this may be better still (less code but perhaps less readable - this is a matter of opinion):
def mod(a, b)
"The remainder of #{a} divided by #{b} is #{a % b}."
end

James Tierney
6,940 PointsHi Andrew, thanks for all the info. It makes it interesting, so many variations on a small segment of code. No doubt I will be seeking further wisdom in the future.
Cheers,
James