Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial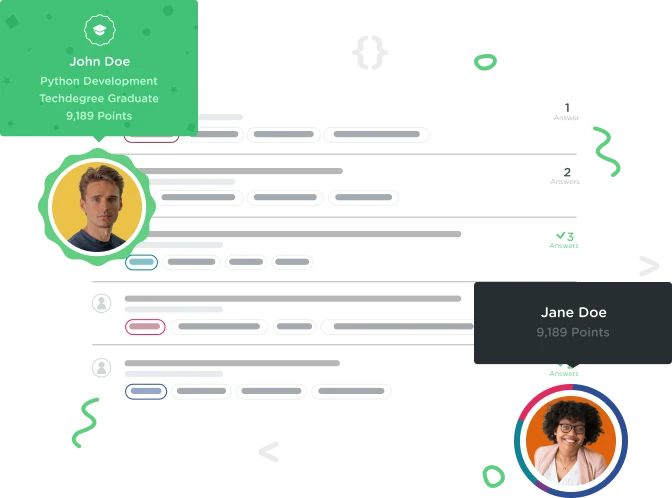
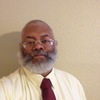
Adiv Abramson
6,919 PointsI created the word_count() function with no errors but test complains "where's word_count()?"
I wrote the following code (developed using IDLE and the pasted into the test input screen:
def word_count(my_phrase):
# convert to lowercase
my_phrase = my_phrase.lower()
# create a list version
my_phrase_list = my_phrase.split(' ')
#remove punctuation
punct_list = ["'", '.', ';', '!', '-', '?', '/']
for each_word in my_phrase_list:
if each_word[-1] in punct_list:
word_pos = my_phrase_list.index(each_word)
my_phrase_list[word_pos] = each_word[: len(each_word)-1]
# add items to phrase_dict: lowercase word as key and 0 as value for initial word count
for each_word in my_phrase_list:
phrase_dict.update({each_word: 0})
# count number of occurrences of each key in dict
for each_key in phrase_dict:
count_of_word = 0
for each_word in my_phrase_list:
if each_key == each_word:
count_of_word +=1
phrase_dict.update({each_key: count_of_word})
return phrase_dict
2 Answers
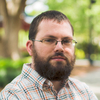
Kenneth Love
Treehouse Guest TeacherIf IDLE isn't complaining about any of that code, I'd ditch IDLE.
You update a phrase_dict
variable that's never created. And your comments should be indented along with the rest of your code.
That's also way more code than you need to pass this code challenge.
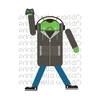
Stephen Bone
12,359 PointsHi Adiv
The error I got when I put your code in workspaces was just that phrase_dict is undefined so I just created an empty dict at the top of the function as in below:
phase_dict = {}
Hope it helps!
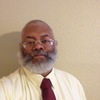
Adiv Abramson
6,919 PointsYep. You are correct. I had created an empty dict object outside of the function while developing the code in IDLE but forgot to relocate it within the function before pasting the code into the test input window. Thanks for your help!
Adiv Abramson
6,919 PointsAdiv Abramson
6,919 PointsIn IDLE I had written the code in a py file. Above the function definition I had the statement
phrase_dict = {}
I forgot to move that into the function definition. I ran the Check Module (Alt+X) command many times while writing the code and it flagged bad indenting, which I fixed. Before pasting the code into the test input screen, I had no indentation errors or any syntax errors. Perhaps the spacing gets thrown off when pasting from one program to another?
I wasn't sure about the placement of the # comment markers. I thought they had to be in the first column. Thanks for providing that clarification.
After reexamining my code I see that I should have outdented everything from the second for loop and beyond. They should not have been nested inside the top for loop.
When I run the code in IDLE using 'I am that I am' for input I get the following result: {'i': 2, 'am': 2, 'that': 1}
I thought adding code to remove punctuation would be advisable since those characters are not part of a word and if you have an input string like 'I am that I am.' with a period, the two "am's" would be treated as separate words, I believe.
I will have to try to find a more succinct way of solving this code challenge if I can. It's fun to learn even though I'm making a ton of errors. :-) Thank you for your help!
Kenneth Love
Treehouse Guest TeacherKenneth Love
Treehouse Guest TeacherRemoving the punctuation is fine, just not required for this code challenge :) I like seeing students thinking outside of the prescribed box, though!