Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial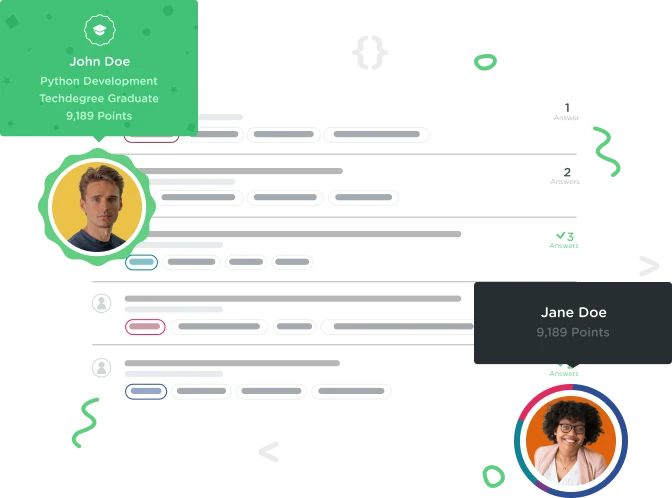
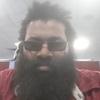
Christopher Sea
3,726 PointsI did the challenge correctly, but I still have questions.
First off, here is my code:
function getRandomNumber(upper, lower) {
var lowNum = parseInt(prompt('Please choose a number.'));
var highNum = parseInt(prompt('Please choose a number larger than ' + lowNum + '.'));
if ( isNaN(lowNum) || isNaN(highNum) ) {
throw new Error('WRONG. \ TRY AGAIN.');
} return Math.floor(Math.random() * (highNum - lowNum + 1)) + lowNum;
}
console.log(getRandomNumber());
- Why is "else" not needed before "return" if it's used in an if statement?
- Are there any other instances where "else" can be supplemented with another statement?
- Since I'm having the user enter in the numbers used for the range, is there a way to re-prompt after the error message appears?
- Would I have to change the error to an alert (or just use console.log), before being able to re-prompt?
- Maybe a stupid question, but why doesn't a number inside quote marks ('5' or "5") work when entered in the prompt?
1 Answer

andren
28,558 PointsElse is only needed if you want some code to only run if the code within the if statement is not run. However in this case since the code within the if block throws an error the code after the if statement will only run if the if block is not run anyway, because of the fact that throwing an error stops the execution of the function. The return statement will therefore never run if an error is thrown, you could add an else statement but it would be entirely redundant.
As mentioned above else is not a mandatory part of if statements, it's only used when you want some code to only execute if the if statement did not run, but if the contents of the if statement causes the rest of the code to not execute, which is mainly the case with return statements and throw error statements then you get the same effect as an else statement without actually needing to use it.
The easiest way to re-prompt would be to use a loop, but I don't think that has been covered in this course yet, I'll include an example showing how to re-prompt below but it will likely not be super clear how it works before you learn more about loops.
Since throwing an error causes the function to stop executing entirely you would indeed have to change the throw error statement to something else in a scenario where you wanted to re-prompt the user.
Quote marks is used to denote a string when you are typing JavaScript code, but when you enter something into a prompt it is treated as a string automatically, so adding quote marks results in a string that actually contains quotes, and since parseInt only converts pure numbers in a string to an int it wont work. Because "1" is not a pure number as it contains a symbol that is not a valid number, namely the quote mark.
Re-prompting example:
function getRandomNumber(upper, lower) {
while(true) { // Start loop which runs until it's manually stopped or the function stops executing
var lowNum = parseInt(prompt('Please choose a number.'));
var highNum = parseInt(prompt('Please choose a number larger than ' + lowNum + '.'));
if ( isNaN(lowNum) || isNaN(highNum) ) {
alert("You need to enter a valid number!");
continue; // Restart loop
} return Math.floor(Math.random() * (highNum - lowNum + 1)) + lowNum;
// Return stops execution of the function, which makes the loop stop as well.
}
}
If you have further questions, or would like some clarification on something I said that might have came across as confusing. Then feel free to ask follow up questions, I'll be happy to clarify anything I can.