Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial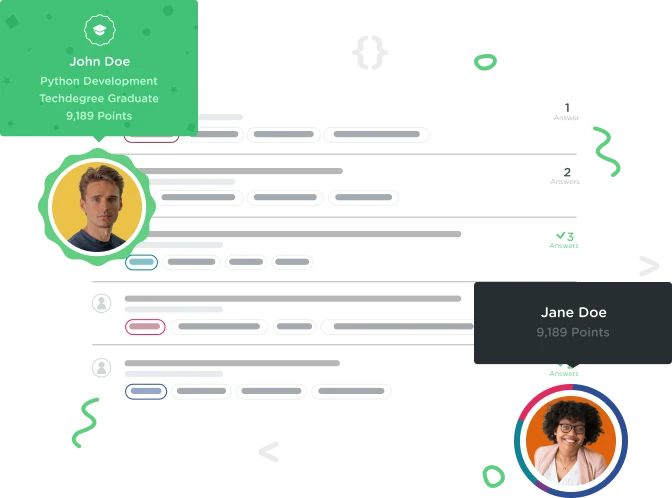

MATTHEW AKROYD
3,982 PointsI don't know how to go about turning this into a dict
I've gotten the program to count how many times the word is in the string, but now I'm not sure how to make it into a dictionary
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(arg):
#something to iterate through string
new_list = arg.split()
#count how many times a word is in the string
for item in new_list:
print item
print(item, new_list.count(item.lower()) + 1)
word_count("I do not like it Sam I Am")
2 Answers
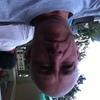
Stuart McIntosh
Python Web Development Techdegree Graduate 22,874 PointsHi there, pointers below
- Create a dict to return word_dict ={}
- l would use lower() after the split, just in case:
new_list = arg.split().lower()
- you are on the right track with count
instances = new_list.count(item)
- Update the dict with the item and instances
word_dict.update({item:instances})
Hope that helps

Wade Williams
24,476 PointsI would do the following:
Lowercase the entire string from the beginning
words = arg.lower().split()
Declare an empty dictionary to hold your word counts
You'll add words to the dictionary using word_dict[word] = value
word_dict = {}
Keep a running total of word counts
Unless you're supposed to use the count method I would just keep a running total. The reason being that the count method goes through the entire list every time you run it, so for "I do not like it Sam I Am" you'll run through the string 8 times with the count method or just 1 time keeping a running total.
All together now:
def word_count(string):
words = string.lower().split()
word_dict = {}
for word in words:
if word in word_dict:
# Word in dict, add one to count
word_dict[word] += 1
else:
# Word not in dict, add it and set it to 1
word_dict[word] = 1
return word_dict