Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial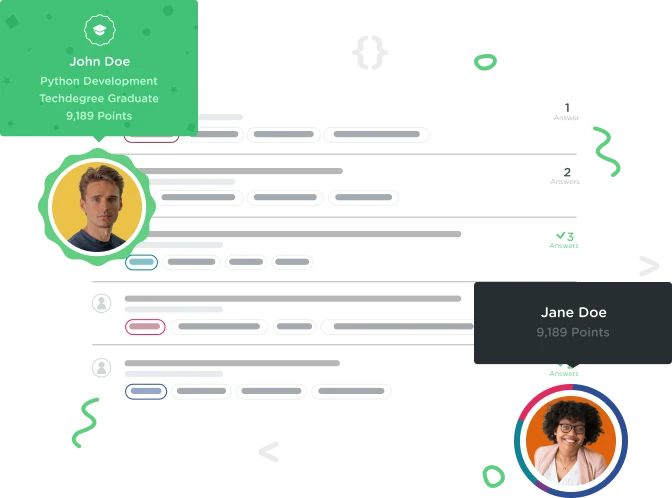

Enya Do
552 PointsI don't know how to solve this assignment.
how do I use the float(). Earlier in my code I tried typing float(a) float(b) right before return.
def add(a, b):
return(a+b)
c = add(5, 7)
5 Answers

Keli'i Martin
8,227 PointsYou can use float()
on a
and b
as you're adding them and returning the result. It should look something like this:
return float(a) + float(b)
Hope this helps.
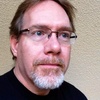
Chris Freeman
Treehouse Moderator 68,454 PointsHere is a walk-through of the challenge. For Task 2 and 3, two solutions are provided, a step-by-step version and a compressed version.
# Task 1 of 3
# I need you to make a new function, 'add'
# 'add' should take two arguments,...
def add(num1, num2):
# add them together, and return the total.
return num1 + num2
# Task 2 of 3
def add(num1, num2):
# Let's make sure we're always working with floats. Convert your
# arguments to floats before you add them together. You can do
# this with the float() function.
fl_num1 = float(num1)
fl_num2 = float(num2)
return fl_num1 + fl_num2
# Task 2 (compressed)
def add(num1, num2):
# Coversion, add, and return
return float(num1) + float(num2)
# Task 3 of 3
def add(num1, num2):
# You're doing great! Just one more task but it's a bigger one.
# Right now, we turn everything into a float. That's great so long
# as we're getting numbers or numbers as a string. We should handle
# cases where we get a non-number, though.
# Add a 'try' block before where you turn your arguments into floats.
try:
fl_num1 = float(num1)
fl_num2 = float(num2)
# Then add an 'except' to catch the possible 'ValueError'.
except ValueError:
# Inside the 'except' block, return 'None'.
return None
# If you're following the structure from the videos, add an 'else:'
# for your final return of the added floats.
else:
return fl_num1 + fl_num2
# Task 3 (commpressed)
def add(num1, num2):
try:
# try the coversion, add, and return, if successful
return float(num1) + float(num2)
except ValueError:
# return None if not sucessful
return None
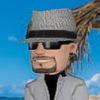
Thomas Beaudry
29,084 PointsChris, that was an awesome explanation for the code challenge! Thank you so much for going into such great detail. I was lost until I read your walk-through! :)

Carlos Quevedo
5,911 PointsHi Chris,
I'm having an issue with Task 3 although my code looks similar to the one you posted. Am I doing something wrong?:
def add(num1, num2):
try:
a = float(num1)
b = float(num2)
except ValueError:
return None
else:
return(a + b)
This is return a "Try Again!" error. Thanks!
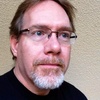
Chris Freeman
Treehouse Moderator 68,454 PointsThe code of the try
block needs indenting
def add(num1, num2):
try:
a = float(num1) # <-- indent
b = float(num2) # <-- indent
except ValueError:
return None
else:
return(a + b)

Carlos Quevedo
5,911 Points*facepalm*It's always the simplest of errors. Thanks again Chris!

Venu karanam
1,484 Pointsdef add(num1, num2): try: a = float(num1) #indentation is very much important b = float(num2) #indentation is very much important except ValueError: return None else: return(a + b)
Enya Do
552 PointsEnya Do
552 PointsThanks! That was really helpful. I was able to complete the challenge.