Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial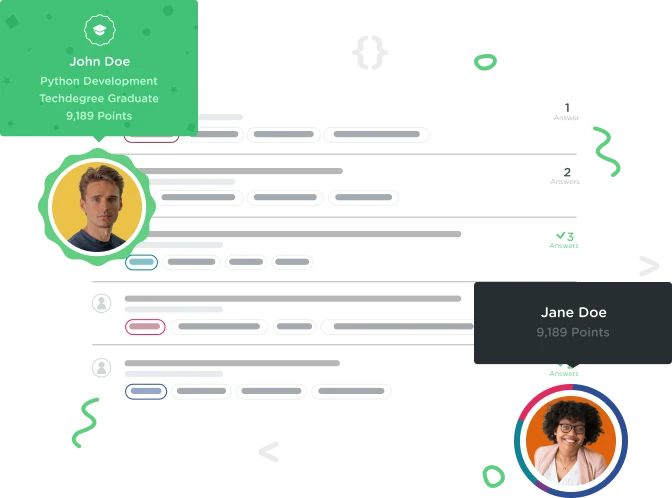

geehun seng
1,705 Pointsi don't know the answer of this question.
Please help me.
var money = 9;
var today = 'Sunday'
if ( money >= 100 || today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 || today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 || today === 'Friday' ) {
alert("Time for a movie");
} else if ( today !== 'Friday' || money ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
1 Answer
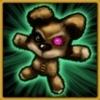
Dave Harker
Courses Plus Student 15,510 PointsHi Geehun,
This challenge is testing the logic so the first condition is evaluating:
'do I have 100 or more?' (FALSE - there is only 9) OR 'is it Friday?' (TRUE - it is Friday!)
An 'OR' condition will be true if any of the conditions being evaluated return true. Because of this, the script as is will always enter this section of code and show the alert "Time to go to the theater"
To fix this logic so we achieve the desired outcome, just change the OR operator to an AND operator
'do I have more than 100?' (FALSE) AND 'is it Friday?' (TRUE)
Because this is an AND logical operator, both would need to be true to execute this section of the script, and so we move on. This pattern remains constant as we move down the script so change all the OR operators ( || ) to AND operators ( && )
The last else if is evaluating:
'Is today NOT Friday?' (but it is Friday, so we would have skipped this which isn't good because this is the outcome we need as Friday is movie day! Sadly they have less than the price of a movie ticket, so no movie for them!)
To fix this logic so we achieve the desired outcome, just change !== (not equal) to === (exactly equals)
So now with appropriate logic in place we reach the desired outcome based on the information given.
I'll paste in the code for you too, just in case there is any confusion :)
var money = 9;
var today = 'Friday'
if ( money >= 100 && today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 && today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 && today === 'Friday' ) {
alert("Time for a movie");
} else if ( today === 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
Hope that helps you out and good luck,
Dave.