Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial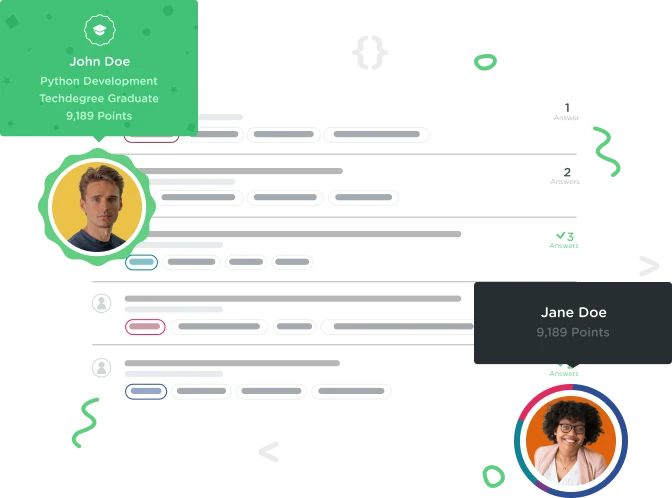

Sirin Srikier
7,671 PointsI don't know what I'm doing wrong. Can someone explain to me the else statement for this challenge?
I tried a few different options for the else statement and I am still getting them wrong. Please help
var isAdmin = false;
var isStudent = false;
if ( isAdmin ) {
alert('Welcome administrator');
} else if (isStudent) {
alert('Welcome student');
} else (isAdmin === isStudent){
alert("Who are you?");
}
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
3 Answers

Brian Fallon
4,733 PointsWith an else statement in any programming language, you are never checking for a conditional. Your first if checks to isAdmin variable, the else if checks the isStudent variable. If both of those are false then you don't know who the person is and you don't pass a third argument.
if ( isAdmin ) {
alert('Welcome administrator');
} else if (isStudent) {
alert('Welcome student');
} else {
alert("Who are you?");
}
This code is what you are looking for. Just to reiterate what I said earlier, you are already checking the only possible options for those 2 variables, therefore no third conditional needs to be checked. If you were checking something else about the variables then you would use an 'else if' statement rather than an 'else' statement.
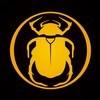
rydavim
18,814 PointsElse is the catch-all clause, so you don't need to evaluate anything. Think of it as saying, "Okay, none of my tests came back as true, so for every other case - do this."
if ( isAdmin ) {
alert('Welcome administrator');
} else if (isStudent) {
alert('Welcome student');
} else (isAdmin === isStudent){
alert("Who are you?");
}
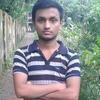
Niyamat Almass
8,176 PointsHi Sirin Srikier you else statement is wrong.The right code is
var isAdmin = false;
var isStudent = false;
if ( isAdmin ) {
alert('Welcome administrator')
} else if (isStudent) {
alert('Welcome student')
} else {
alert("Who are you?");
}