Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial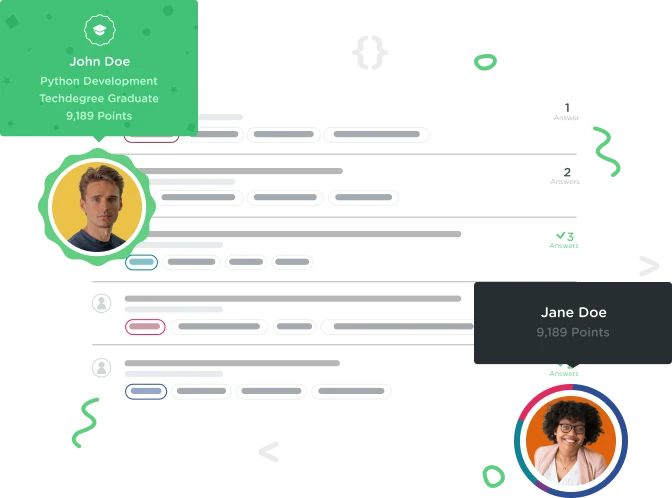
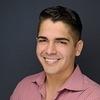
ian izaguirre
3,220 PointsI don't know why the Math in my code is not giving random answers?
I put it in an 'upper' number and a 'lower' number in my prompt and get the wrong final answer? Whats wrong with my Math, I can't notice it being any different then the videos math formula ?
When the code runs, if I type in the prompt 'upper' 7 and in the prompt 'lower' 4 , my answers are always either 4 or 7. There is no variation. So there is no randomness, since the answers are always the upper or lower prompt input ?
// Function accepts a lower and an upper value and produces a value between them
function getMyNumber(upper, lower) {
upper = parseInt(prompt('Please Input Your Upper Number'));
lower = parseInt(prompt('Please Input Your lower Number'));
return Math.floor(Math.random() * (upper - lower + 1)) + lower;
}
alert( getMyNumber() );
```
6 Answers
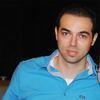
Ezra Siton
12,644 PointsFirst this is not the example from the video (you add prompt).
Problem 1 - cast value: promt return a string. So you must cast the value in your example
Please note that result is a string. That means you should sometimes cast the value given by the user. For example, if his answer should be a Number, you should cast the value to Number. var aNumber = Number(window.prompt("Type a number", "")); (MDN)
https://developer.mozilla.org/en-US/docs/Web/API/Window/prompt
Problem 2 - bad practice Your code structure is wrong (not syntax error only bad practice). Its not make sense to call a function getRandomNumber(4,2) - and than inside the function override this parameters with promt (If this is the case use function without parameters)
Fix 1+2:
function getRandomNumber(lower, upper){
return Math.floor(Math.random() * (upper - lower + 1)) + lower;
}
upper = Number(prompt('Please Input Your Upper Number'));
lower = Number(prompt('Please Input Your lower Number'));
getRandomNumber(upper, lower);
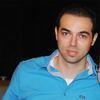
Ezra Siton
12,644 PointsNAN (Not-a-Number). You trying to alert the function but you dont send any values to this function.
function getMyNumber(upper, lower) {
return Math.floor(Math.random() * (upper - lower + 1)) + lower;
}
var questionOne = parseInt(prompt('Please Input Your Upper Number'));
var questionTwo = parseInt(prompt('Please Input Your Lower Number'));
// correct code
alert (getMyNumber(questionOne,questionTwo));
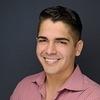
ian izaguirre
3,220 PointsI see, just noticed that I forgot to assign the getMyNumber a variable when I passed new parameters and then the variable should have been in the alert, like this:
// Function accepts a lower and an upper value and produces a value between them
function getMyNumber(upper, lower) {
return Math.floor(Math.random() * (upper - lower + 1)) + lower;
}
var questionOne = parseInt(prompt('Please Input Your Upper Number'));
var questionTwo = parseInt(prompt('Please Input Your Lower Number'));
var randomCalculation = getMyNumber(questionOne,questionTwo);
alert( randomCalculation );
'''
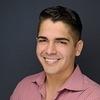
ian izaguirre
3,220 PointsThank you for saying "It makes no sense to call a function with parameters and override the parameters inside the same function"
I did not catch that beforehand.
So I rewrote it but my final alert keeps returning "NaN", and I do not understand why it thinks something is not a number?
// Function accepts a lower and an upper value and produces a value between them
function getMyNumber(upper, lower) {
return Math.floor(Math.random() * (upper - lower + 1)) + lower;
}
var questionOne = parseInt(prompt('Please Input Your Upper Number'));
var questionTwo = parseInt(prompt('Please Input Your Lower Number'));
getMyNumber(questionOne,questionTwo);
alert( getMyNumber() );
'''
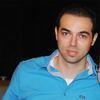
Ezra Siton
12,644 PointsYes. your last code example works fine.
OUT OF TOPIC: learn how to markdown code her https://teamtreehouse.com/library/code
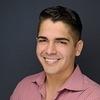
ian izaguirre
3,220 PointsThank you I am going to watch that video right now. Thank you for taking the time to help break this down for me, I understand my errors now, I really appreciate it!
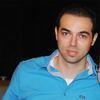
Ezra Siton
12,644 PointsYou welcome :)

Rudy Soo
Full Stack JavaScript Techdegree Student 1,468 PointsYou'll need to use an two argument value and send it to two parameter . So you don't need to use prompt() to ask a number value
ian izaguirre
3,220 Pointsian izaguirre
3,220 PointsI changed my question and when I submitted the edit you answered, pure chance. So I just updated my question since I realized the alert and prompt turn numbers into strings problem. I will read your answer and see if it answers my new edit. Also I know I added prompt and the video did not.