Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial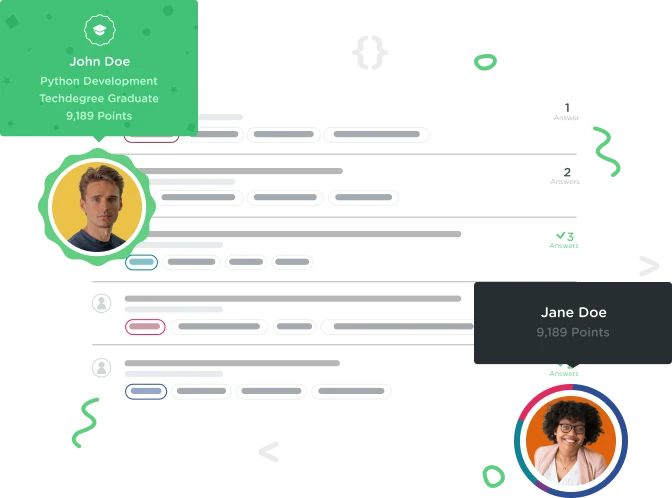
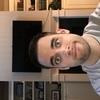
Daniel McFarlin
5,168 PointsI don't quite get the "else:" part of the quiz. Please help!
The last part of this quiz is not really making any sense to me. Please help! I am thinking I just don't quite get it because of the wording, but not positive.
def add(x, y):
try:
return float(x) + float(y)
except ValueError:
return None
else:
return float(x) + float(y)
2 Answers
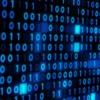
Alexander Davison
65,469 PointsYour code is actually correct.
Python is just picky about indentation, so Python spits an error out at you because you didn't add the space before every line of code except the line where you are defining the function.
# Python spits an error at you because you didn't use enough indentation (spacing)
def add(x, y):
try:
return float(x) + float(y)
except ValueError:
return None
else:
return float(x) + float(y)
# Python doesn't throw an error because there's proper indentation
def add(x, y):
try:
return float(x) + float(y)
except ValueError:
return None
else:
return float(x) + float(y)
Still, you can simplify your code by getting rid of the else
. It isn't required in this situation, because if the code in the try
doesn't cause an error, it is going to return it anyway.
Keep in mind that the code in the try
is actually run by Python, it isn't just tested to see if there's an error in there!
Final solution:
def add(x, y):
try:
return float(x) + float(y)
except ValueError:
return None
I hope this helps. ~Alex
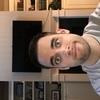
Daniel McFarlin
5,168 Pointsfacepalm
Thank you so much Alex! I was stuck on that forever! haha my code was right, but the indention was just slightly off. It worked!
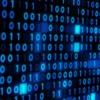
Alexander Davison
65,469 PointsLol no problem :)