Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial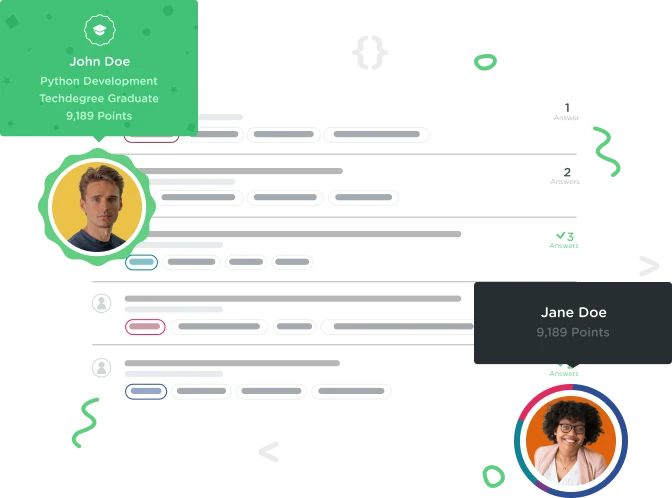

Nathalie Agnekil
6,665 PointsI don't understand any of this...
Can someone explain this further to me? I get the loop and understand how it works.
Where is the name "list" coming from and what does it contain? I mean, how can they use list.length, when there is no array called "list"?
What is the "print()" thing? I google it and it says there is no such thing as print() in JavaScript...
Using the html-tags.. I don't get the connection... Does it somehow works as id and classes in html+css? That the <ol> now behaves that way if you use it in the HTML just like the ol can look a certain way if you give it values in css? What's happening here? How is it printing the list when the index.html-file does not contain a list? Is using html-tags as strings like using html-tags in html-files?
I'M SO CONFUSED and don't feel like anyone can answer these questions for me to understand. Can you please dumb it down for me to a level of a 5-year-old?
4 Answers
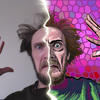
Seth Johnson
15,200 PointsI've also been staring at this particular video for at least an hour trying to wrap my brain around it; in a lot of ways it builds off of a lot of the stuff already covered in the "JavaScript Basics" course that I was having trouble recalling on account of so much information flying at me so quickly.
Basically the way I see it is, after having taken dozens of pages of notes, IMHO pretty much every single term, keyword, concept, and so on needs some kind of quotation marks or a parentheses or an asterisk or SOMETHING that helps further explain it. Here's what I came up with for this section in particular.
You'll note the heavy use of quotation marks and exclamation points...this is entirely me yelling at myself so I apologize if it seems aggressive. : D
var playList = [
'I Did It My Way',
'Respect',
'Imagine',
'Born to Run',
'Louie Louie',
'Maybellene'
];
Here is where confusion can start to occur!
function print(message) {
document.write(message);
}
The word "message" used in the () for the "print" function seen above is simply a parameter, which means it's not necessarily used for anything besides being a "placeholder" word that gets replaced with any information "sent to" the "print" function each subsequent time it's called!
In this example, it's the "listHTML" variable that's nested inside the "printList" function (which can be seen in the third part of example code)!
...Doesn't take long to get to the point where even MORE confusion can occur!
function printList( list ) {
var listHTML = '<ol>';
for ( var i = 0; i < list.length; i += 1 ) {
listHTML += '<li>' + list[i] + '</li>';
}
listHTML += '</ol>';
print(listHTML);
}
printList(playList);
The word "list" used in the () for the "printList" function seen above is simply a parameter, which means it's not necessarily used for anything besides being a "placeholder" word that gets replaced with any information "sent to" the "printList" function each subsequent time it's called! In this example, it's the "playList" array that was declared at the very beginning of this JS code!
Since the array is in the parantheses directly following the called "printList" function on the last line, the information in the "playList" array is "sent to" to "printList" function, which then follows the "for" loop that builds the ordered HTML list that appears on the page!
Hope this helps! (If anyone wants to jump in & let me know I'm completely wrong here, please let me know.)
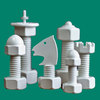
Steven Parker
241,957 PointsI assume the "list" you are talking about is the parameter of the "printList" function that is defined starting on line 14 of "playlist.js". A parameter name is just a placeholder for the argument that will be passed in when the function is actually called. On line 22 it is called with the argument "playList", and "playList" is defined starting on line 1.
At about 5:06 in the video, you can see where "print()" is defined starting on line 10 of "playlist.js".
The "index.html" file does not contain the list. As I mentioned already, the list is the first thing in "playlist.js". The "printList" function combines the list with some HTML tags to make it look nice, and then adds it to the page.

Trisha Mitobe
Front End Web Development Techdegree Student 6,844 PointsThanks Seth for the very thorough response. I was confused by the placeholder as well...crystal clear now.
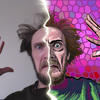
Seth Johnson
15,200 PointsNo problem!

pat barosy
6,759 PointsCan someone explain the relationship between the print & printList functions? It seems that the print function is called inside of the printList function. If we were to do them separately, what would print output & what would printList output?
Thank you
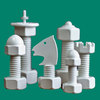
Steven Parker
241,957 PointsThey work on different kinds of arguments. "Print" takes a string and puts it on the page, but "printList" takes an array and converts it into an HTML unordered list while using "print" to display the items.
Thomas Grimes
10,726 PointsThomas Grimes
10,726 PointsRegarding the parameter name "list", you are correct. It is serving as a placeholder in the function Dave created in the video. Then at the bottom he actually calls the function we created and you can see he passes the array named "playList" (the list of song titles.) To test this, you can change all instances of the word "list" in your function to anything you like. For example, the following, where I have replaced instances of "list" with "FOO", will work just as well as what Dave created.
Alec Hawley
13,346 PointsAlec Hawley
13,346 PointsYou are a lifesaver. Thank you so much. I'm in somewhat of a coding bootcamp, and I have to get this done quickly. This particular video haunted me for nearly a two days.