Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial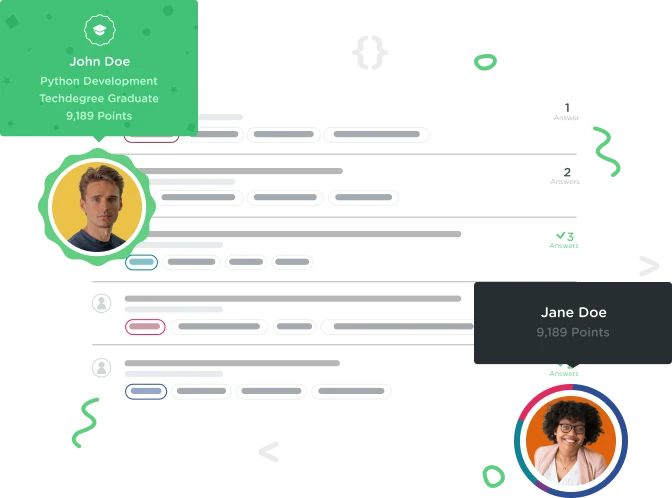

Michael Partridge
7,129 PointsI don't understand how "ben" is getting stored in the string resources file under the key we made.
Is the putExtra() method pushing the user inputted name to the resources file? From what I understand all we have defined in the string resource file is equal to: String key_name = "name";
1 Answer
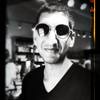
Taha Abbasi
12,865 PointsThis is going to be a bit of a lengthy explanation, but don't get overwhelmed. I went into a lot of detail, and tried to break down what is happening. Just read through it step by step and it should make sense. Let me know if you have any questions. Here goes nothing...
The answer to your question is actually in MainActivity.java
We aren't storing "ben" per say in the String Resource file. Take a look at the code below to see what's happening:
in MainActivity.java we are declaring a String variable "name" and retrieving the user's name that they input as below:
String name = mNameField.getText().toString();
Then we are passing this variable to the method we created called startStory. The startStory method takes a parameter of a String name. This is where we are passing the name we just retrieved into this method.
// Calling the method to move to StoryActivity and passing in the name we retrieved.
startStory(name);
Now if you follow just the above explanation, So far:
- We know we retrieved the name that the user entered
- We passed that name to a method called startStory
But Why???? Let me explain by going over that startStory method and why it needed the name input, and what it is doing with that input.
So the startStory method goes like this:
private void startStory(String name) {
Intent intent = new Intent(this, StoryActivity.class);
intent.putExtra(getString(R.string.key_name), name);
startActivity(intent);
}
in the above code we are first creating a new Intent object called intent. This the first step to passing information from one Activity to another. Here we are passing information form our MainAcitivity.java to StoryActivity.java so first we created the intent object with the statement:
Intent intent = new Intent(this, StoryActivity.class);
Now notice that at object creation we passed in (this, StoryActivity.class). This is because the object takes 2 parameters in this case. 1st the class we want to pass information from, 2nd the class we want to pass information too.
Next, we have the intent object created, now we have to decide what data we want to pass. We do this by using a method for the intent object called putExtra. This method takes two parameters, (String key, String value). So whatever we put in here can be pulled out in the other class by looking for the key. It acts kind of like a dictionary. Here is how we wrote this line of code:
intent.putExtra(getString(R.string.key_name), name);
// complete the code related to the intent by writing the following line of code:
startActivity(intent);
so the line to pay attention to is
intent.putExtra(getString(R.string.key_name), name);
The key here is R.string.key_name and the value is name which was the name we passed in earlier when we called the method.
When you look at how we pull this data out in the StoryActivity.java you will see why string resources are important.
In StoryActivity.java
after creating a new Intent object we retrieved the name that was passed onto this class form the previous class as below:
String name = intent.getStringExtra(getString(R.string.key_name));
using the getStringExtra method on the Intent object. The getStringExtra method takes a key and uses it to find the value for that key. It has to match what was used in the putExtra method otherwise it causes a fatal error. If we did not use stringResources we would have used the putExtra and getStringExtra methods as below:
// Passing class PutExtra method
intent.putExtra("name", name);
// Receiving class GetExtra method
intent.getStringExtra("name");
This is prone to error because if we used the key "name" in the put and used the key "Name" in the get it will cause a fatal error. Thus we use StringResources so we can declare one key name and use it for put and get.
In our cases we decided to use the below:
<string name="key_name">name</string>
where the name of the "key" is "key_name" and the value is: name and it is of type string.
and thus we can use the below code without typing the key, instead using the string resource.
// ManActivity code
.....
mStartButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String name = mNameField.getText().toString();
// Calling the method to move to StoryActivity
startStory(name);
}
});
//Toast.makeText(this, (CharSequence) mNameField, Toast.LENGTH_SHORT);
}
private void startStory(String name) {
Intent intent = new Intent(this, StoryActivity.class);
intent.putExtra(getString(R.string.key_name), name);
startActivity(intent);
}
.....
// StoryActivity code
.....
Intent intent = getIntent();
String name = intent.getStringExtra(getString(R.string.key_name));
if (name == null) {
name = "No Name Passed In";
}
Log.d(TAG, name);
}
...
Michael Partridge
7,129 PointsMichael Partridge
7,129 PointsVery good explanation. I really appreciate the time you took to write out this answer so clearly. I have a much better grasp on the subject now. I did notice a few typos and capitalization errors I suggest you fix because I'm sure I'm not the only one who is going to find your post very helpful.
Now just to be clear:
This line
intent.putExtra(getString(R.string.key_name), name);
is changing the string value of "key_name" in this line
<string name="key_name">name</string>
from "name" and replacing it with the string value of the variable name used in the first line I referenced. That value now becomes retrievable and we retrieve and store it with:
String name = intent.getStringExtra(getString(R.string.key_name));
P.S. How did you get your blocks of code to show up like that?
Taha Abbasi
12,865 PointsTaha Abbasi
12,865 PointsHi Michael,
Sorry about the typos. First lets go over the code blocks, I had the same issue when I was trying to post questions, turns out any code line can be wrapped in three ` This is the key on the left of 1 on your keyboard. The other symbol on this key in the keyboard is ~. Check out the "Tips for asking questions" video on the right of this page. That has more detail about this.
Now about your question:
Now just to be clear:
This line
intent.putExtra(getString(R.string.key_name), name);
is changing the string value of "key_name" in this line
<string name="key_name">name</string>
from "name" and replacing it with the string value of the variable name used in the first line I referenced. That value now becomes retrievable and we retrieve and store it with:
String name = intent.getStringExtra(getString(R.string.key_name));
Actually
Let me explain with some examples:
This line
intent.putExtra(getString(R.string.key_name), name);
So in the above here is a generic requirement
intent.putExtra(key, value);
When we type
intent.putExtra(getString(R.string.key_name), name);
it changes to
intent.putExtra("name", name);
remember the generic requirement
intent.putExtra(key, value);
So for this extra object now the key is "name" and the value is the variable name, which holds the value that we got from the user input in this line of code.
String name = intent.getStringExtra(getString(R.string.key_name));
Thus the below is actually not happening: rather what I explained above is. Let me know if you have questions.
is changing the string value of "key_name" in this line
<string name="key_name">name</string>
So in the above here is a generic requirement
<string name =key>value</string>
from "name" and replacing it with the string value of the variable name used in the first line I referenced. That value now becomes retrievable and we retrieve and store it with:
String name = intent.getStringExtra(getString(R.string.key_name));
P.S. Sorry about the typos or Capitalization errors