Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial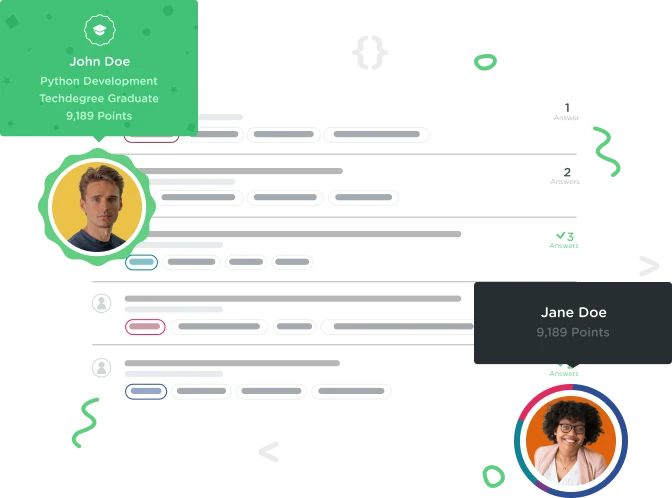

Hope Mallary
900 PointsI don't understand refactoring.
I have added the main function and tabbed everything in so that it is inside the new main funcion. help
def show_help():
# print out instructions on how to use the app
print("What should we pick up at the store?")
print("""
Enter 'DONE' to stop adding items.
Enter 'HELP' for this help.
Enter 'SHOW' to see your current list.
""")
def show_list(shopping_list):
# print out the list
print("Here's your list:")
for item in shopping_list:
print(item)
def add_to_list(shopping_list, new_item):
# add new items to our list
shopping_list.append(new_item)
print("Added {}. List now has {} items.".format(new_item, len(shopping_list)))
return shopping_list
def main():
show_help()
# make a list to hold onto our items
shopping_list = []
while True:
# ask for new items
new_item = input("> ")
# be able to quit the app
if new_item == 'DONE':
break
elif new_item == 'HELP':
show_help()
continue
elif new_item == 'SHOW':
show_list(shopping_list)
continue
add_to_list(shopping_list, new_item)
show_list(shopping_list)
1 Answer

Ryan S
27,276 PointsHi Hope,
Indentation is extremely important in Python. Some languages aren't affected by indents and white space, but in Python if you want to move a bunch of existing code into a function (or a loop, or an if
statement, etc), you have to make sure that everything is indented under the statement in question. The other thing you have to be careful of, is that the code you are indenting still must maintain its relative indentation.
Notice how all of your if's and elif's below the while loop are not actually in the while loop, but they were inside the loop in the original code given to you by the challenge. When you indent the while True:
statement, you also need to make sure you indent the statements below it.
The easiest way through this challenge is to look at the original code you were given. Take note of the indentation and see where everything sits relative to each other. Then make sure it remains exactly the same, except of course that everything will be indented by one tab in order to put it inside the function you've defined.
Good luck.