Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial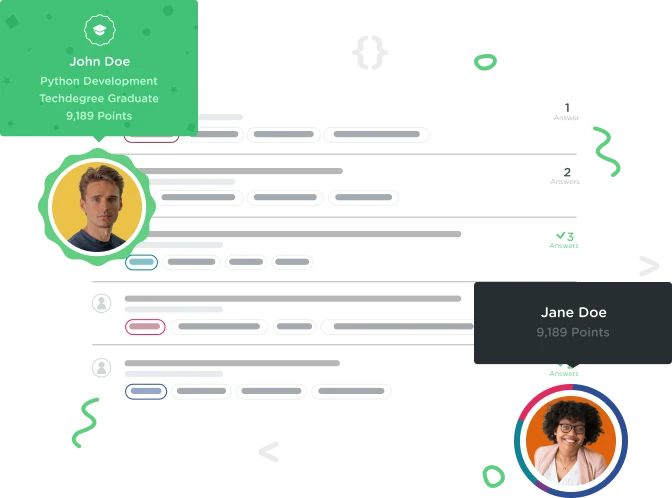

Aaron Hasbrouck
1,295 PointsI don't understand what I'm doing wrong
Hi, I'm really not understanding why I'm getting an error for my array being listed out of order.
var temperatures = [100,90,99,80,70,65,30,10];
var i
function printList( list ){
var listHTML = '<ol>';
for ( i = 0; i < temperatures.length; i += 1){
listHTML += '<li>' + list[i] + '</li>';
}
listHTML += '</ol>';
}
console.log(i);
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
1 Answer
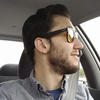
Benjamin Larson
34,055 PointsYou have a lot more going on in your code than the challenge is really looking for in terms of the printList
function and printing HTML list items. These should be removed otherwise the challenge will fail.
The for loop declaration is good, although you could condense things by declaring the i
variable inside of it. You'll need to move your console.log function inside the for loop in order for it to traverse each of the values in the array. Finally, console.log(i)
will only log the index. You need to log the value of the temperature array at that index, so give that a shot.
for (var i = 0; i < temperatures.length; i += 1) {
// add console.log() here
}
Andrew Elliott
3,306 PointsAndrew Elliott
3,306 PointsYour problem is that you are doing your loop in an improper way. Here's what it should look like this: