Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial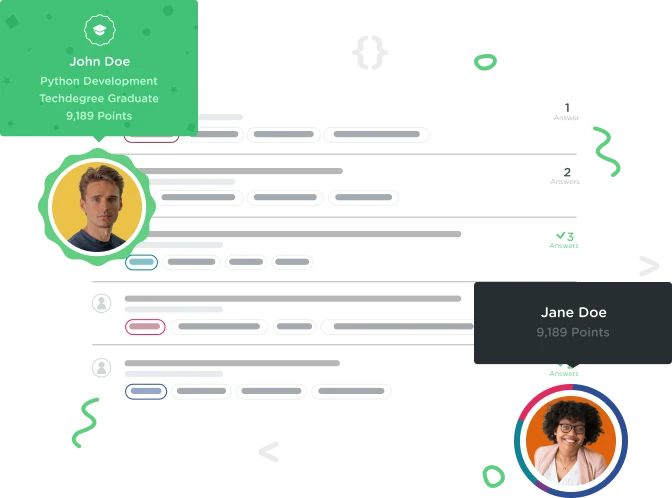

Jason Portilla
15,345 PointsI don't understand what i'm doing wrong in the 3 part of the challenge with exceptions
You're doing great! Just one more task but it's a bigger one.
Right now, we turn everything into a float. That's great so long as we're getting numbers or numbers as a string. We should handle cases where we get a non-number, though.
Add a try block before where you turn your arguments into floats.
Then add an except to catch the possible ValueError. Inside the except block, return None.
If you're following the structure from the videos, add an else: for your final return of the added floats.
def add(num1, num2):
try:
return float(num1) + float(num2)
except ValueError:
return none
else:
print(num1 + num2)

Jason Portilla
15,345 PointsBoris what I understand is under the try: block, it converts num1 and num2 into a float. If it a number then it passes and skips the except block and go to the else block. In the else block it prints out the float of num1 + num2. The Except block happens when something other than a number is entered inside the arguments. This returns None which is nothing and the program ends.

Gary Gibson
5,011 PointsI think the else should be followed by return(float(num1) + float(num2)), not print(num1 + num2). That's the structure that worked for me. The code asked for "return", not "print." And you have to be sure your arguments are still converted to floats in this part of the block
else:
return(float(num1) + float(num2))

Justin Burnett
3,427 PointsThis one through me for a loop, and even with looking at the answers i'm not sure i'm following the logic.... I think i need to redo this whole course now =\
7 Answers
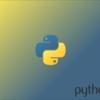
Kevin Faust
15,353 Pointsjust gotta capitalize the n in none at line 5. should be None

Senay Berhe
7,871 PointsI agree.
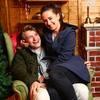
Patric Daniel Pförtner
1,542 PointsHi Jason,
You are very close to the answer, here is how I did it:
def add(num1, num2):
try:
return float(num1) + float(num2)
except ValueError:
return None
else:
print(num1 + num2)
Why are you learning Python? Maybe we have the same aims! I am learning it to bring my Start UP www.wolf-gate.com to the next level. Thank´s to Kenneth Love it´s easily possible :)

Mathew V L
2,910 PointsWhy is the else print?
The exercise says for your final return which is confusing

vijaya inturi
538 Pointsdef add(x, y): try: return float(x) + float(y) except ValueError: return None else: print(x+ y)

nathanielcusano
9,808 Pointsdef add(a, b):
try:
C= float(a) + float(b)
except ValueError:
return None
else:
return C
add("123", 2)
# Basically you need to store the sum in variable C. However, if you call the function
# with something like "zq23" it won't recognize it either as a string nor as an integer and
# will not be able to convert it to a float ,so you will get the value error.
# You could also choose to store float(a) and float(b) in a separate variable like D = float(b)
# In that case, you would want to return C + D
# I called it with "123" and 2 so it gets converted to 123 and the sum is 125. You can check
# this at pythontutor.com to see more clearly
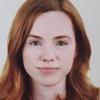
mckbas
14,166 PointsThis is what worked for me:
def add(x, y):
try:
return float(x) + float(y)
except ValueError:
return None
else:
return x + y

sai jayanth kashyap
3,293 Pointsits not working for me. ''' def add(x, y): try: return float(x) + float(y) except ValueError: return None else: return x + y '''
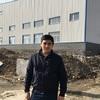
ali mur
UX Design Techdegree Student 23,377 Pointsyou need to indent your code.

daniel escobar
Courses Plus Student 563 PointsFor those who get errors, I highly recommend that you try your code in workspaces because it tells you where your error is. For me, it took me a long time until i figure it out that my issue was indentation. Trust me, try your code in workspaces
Boris Aroche
iOS Development Techdegree Student 555 PointsBoris Aroche
iOS Development Techdegree Student 555 PointsDid you figure this one out? I'm still really confused about challenge 3