Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial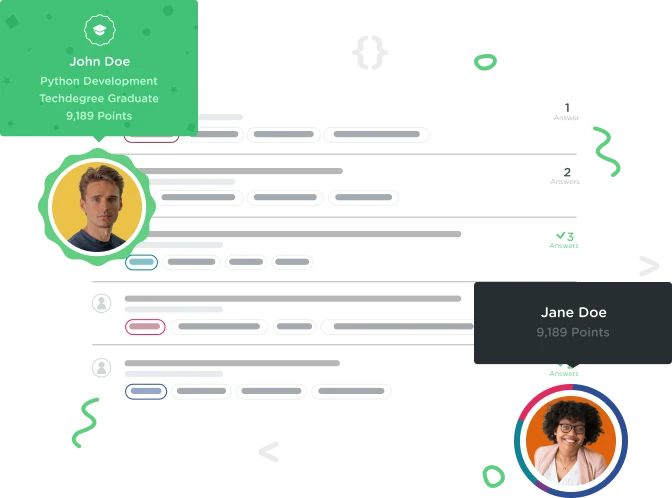
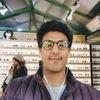
Shreemangal Sethi
Full Stack JavaScript Techdegree Student 15,552 PointsI don't understand where am i wrong in this.
It says respond with a string "Hello" and then by contents of the 'name' URL param. My Code is
get "/greet/:name" do "Hello" page_contents(params[:name]) end
require "sinatra"
get "/greet/:name" do
# YOUR CODE HERE
"Hello"
page_contents(params[:name])
end
3 Answers
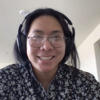
AJ Tran
Treehouse TeacherOK, let's explore what you have some more! By the way, you can enclose your code snippets in triple-backticks so it is formatted for viewing in Markdown. You can put the language name on the first set of backticks so that your code will be highlighted.
```ruby
Let's look at your code again and break it down.
require "sinatra"
get "/greet/:name" do
# YOUR CODE HERE
@name = params[:name]
@content = page_content(@name)
"Hello " + @content
end
First, this challenge does not require you to use the page_content
method. That was just part of a demonstration in a previous video and is not related to this challenge.
require "sinatra"
get "/greet/:name" do
# YOUR CODE HERE
@name = params[:name]
"Hello " + _____
end
In Sinatra, all of your params are a string data type. So you can concatenate "Hello "
with params[:name]
. With your code, it could be modified to look like this and it will pass the challenge:
require "sinatra"
get "/greet/:name" do
# YOUR CODE HERE
@name = params[:name]
"Hello " + @name
end
However, there is no need to declare an instance variable in this code block. This will work as well:
require "sinatra"
get "/greet/:name" do
# YOUR CODE HERE
"Hello " + params[:name]
end
And if you want to be fancy, you can interpolate the string instead of concatenating it:
require "sinatra"
get "/greet/:name" do
# YOUR CODE HERE
"Hello #{params[:name]}"
end
I hope this clears it up for you, Shreemangal Sethi
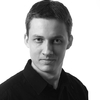
Maciej Czuchnowski
36,441 PointsRight now, since these are two separate lines, the last thing this returns is the page_contents(params[:name])
part. You most likely need something like "Hello name", so your best bet would be string interpolation.
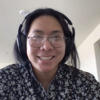
AJ Tran
Treehouse TeacherIn Ruby, there is a concept called Implicit Return. That means that the last line of a method is your return value. Right now you are just returning the name
from params
. The data type of name
should be a string - so you will be able to interpolate or concatenate with "Hello"
to pass the challenge!
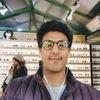
Shreemangal Sethi
Full Stack JavaScript Techdegree Student 15,552 PointsI tried this but it does not work
require "sinatra"
get "/greet/:name" do # YOUR CODE HERE @name = params[:name] @content = page_content(@name) "Hello " + @content end