Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial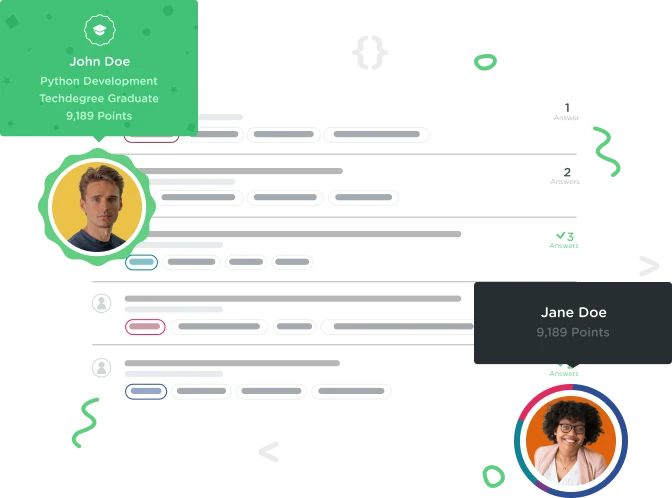

Ana Bedacarratz
1,814 PointsI don't understand why this doesn't work. I have been following the unpacker model from the previous lecture. Thanks!
Let's test unpacking dictionaries in keyword arguments. You've used the string .format() method before to fill in blank placeholders. If you give the placeholder a name, though, like in template below, you fill it in through keyword arguments to .format(), like this: template.format(name="Kenneth", food="tacos") Write a function named string_factory that accepts a list of dictionaries as an argument. Return a new list of strings made by using ** for each dictionary in the list and the template string provided.
# Example:
# values = [{"name": "Michelangelo", "food": "PIZZA"}, {"name": "Garfield", "food": "lasagna"}]
# string_factory(values)
# ["Hi, I'm Michelangelo and I love to eat PIZZA!", "Hi, I'm Garfield and I love to eat lasagna!"]
template = "Hi, I'm {name} and I love to eat {food}!"
values = [{"name": "Michelangelo", "food": "PIZZA"}, {"name": "Garfield", "food": "lasagna"}]
def string_factory(name=None, food=None):
if name and food:
print(template.format(name, food))
else:
print("MISSING DATA")
string_factory(**values)
2 Answers
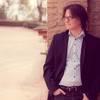
Michal Janek
Front End Web Development Techdegree Graduate 30,654 PointsYour code has a few minor flaws.
- You have to return a list according to the challenge's instructions. Your function is not returning anything it just prints the results. You need a RETURN statement
- The IF condition is not really necessary here but since we have a list of values we do need to iterate through it. We need a FOR loop.
I could use list comprehension but for the sake of clarity I did not.
def string_factory(val):
newList = []
for x in val:
newList.append(template.format(**x))
return(newList)
First we create an empty list
newList = []
Then we will iterate through the list of dictionaries. The list will be passed to us when we call the function. This is the call not the iteration (this is how we get the list into our function).
string_factory(this_is_going_to_be_the_list)
Now back to the inside of the function. During the iteration we unpack each iterated element (dictionary represented by X) and format its key-value pair into template. Then we append it into the list which gets returned.
for x in this_is_going_to_be_the_list:
newList.append(template.format(**x))
return newList

Ana Bedacarratz
1,814 PointsThank you! That makes a lot of sense. I am out of the woods :)
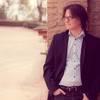
Michal Janek
Front End Web Development Techdegree Graduate 30,654 PointsSure not a problem good luck :)