Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial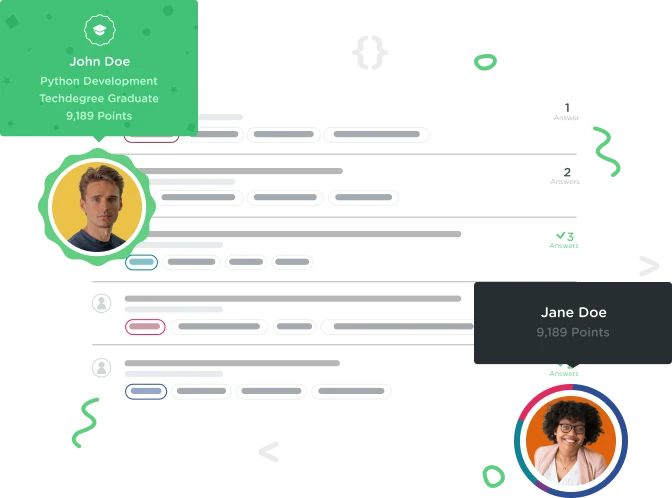

Thomas Smith
23,240 PointsI got the correct output in the python shell, but the website is telling me this is wrong.
def word_count(string): dictionary = {} for word in string.lower().split(" "): if word in dictionary: dictionary.update({word : dictionary[word] + 1}) else: dictionary.update({word : 1}) return dictionary
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(string):
dictionary = {}
for word in string.lower().split(" "):
if word in dictionary:
dictionary.update({word : dictionary[word] + 1})
else:
dictionary.update({word : 1})
return dictionary
2 Answers
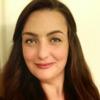
Jennifer Nordell
Treehouse TeacherHi there! Oh wow, you're really close here. And the key to solving this is in the "Bummer!" message. My guess is that your data set is not sending in any other white space like new lines or tabs, but the checker likely is.
Bummer! Hmm, didn't get the expected output. Be sure you're lowercasing the string and splitting on all whitespace!
You must split on all white space, but currently you're only splitting on spaces.
If I change your .split(" ")
to .split()
, your code passes with flying colors! Using the split method with no arguments will indicate that you should split on all white space.
Hope this helps!

oe2
Python Development Techdegree Graduate 14,079 PointsFound this post working on the same challenge. Turns out I dont really understand dictionary. I understand most of this code, I think, but can
t really seem to understand exactly what the code after the if word in dictionary:
How does this line of code give you the exact count? dictionary.update({word : dictionary[word] + 1})
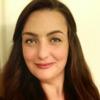
Jennifer Nordell
Treehouse TeacherOk, let's see if I can explain. We start with an empty dictionary. Now we're going to take whatever was sent in and split it on all white space so we're seeing individual words. In the beginning of the for loop we say that we want to take each individual word and look at it after we turn it to lowercase. It's going to take the first word we put in and look at it. When it reaches the if statement, that will immediately fail because our dictionary is empty. There are no words in it. So, obviously, the word is not present... yet. Then it hits the else statement. And it adds that word to the dictionary with a count of 1 because so far we've seen that word one time. It continues going through the string and looking at the individual words. Now, likely as not, it will eventually come to a word that already exists in that dictionary. This is where the if
statement succeeds. And this is the line you seem to be having difficulty with. This line says update the dictionary at this word and increase its count by one. Because we start by adding the word with a count of one, the second time we see it we increase 1 to 2. And the third time we see it, the count goes from 2 to 3 etc. When the loop finishes all words will have been put into the dictionary and the dictionary will have a correct count.
Hope that clarifies things!