Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial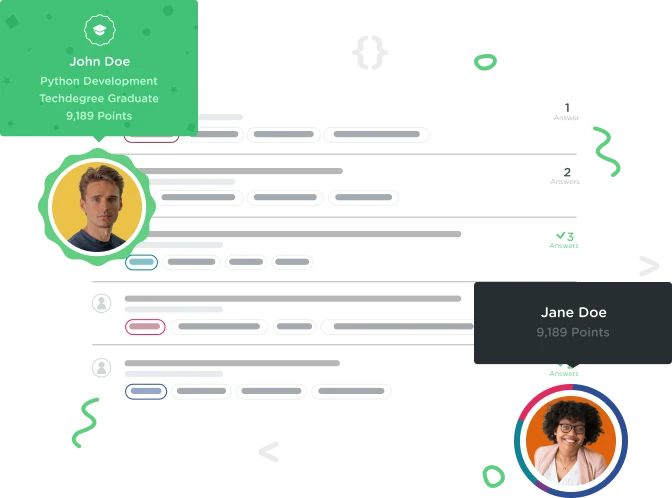

Brandy Fishback
4,223 PointsI have an array and a form. How do I pull randomized questions and answers from that array? Do I use array_rand?
//the form
div class="container">
<div id="quiz-box">
<p class="breadcrumbs">Question # of #</p>
<p class="quiz"><?php echo "What is" . $questions["leftAdder"] . "+" . $questions ["rightAdder"];?></p>
<form action="index.php" method="post">
<input type="hidden" name="id" value="0"/>
<input type="submit" class="btn" name="answer" value="<?php echo $questions ["correctAnswer"];?>" />
<input type="submit" class="btn" name="answer" value="<?php echo $questions ["firstIncorrectAnswer"];?>" />
<input type="submit" class="btn" name="answer" value="<?php echo $questions ["secondIncorrectAnswer"];?>" />
</form>
</div>
//the array
<?php
$questions[] =
[
"leftAdder" => 3,
"rightAdder" => 4,
"correctAnswer" => 7,
"firstIncorrectAnswer" => 8,
"secondIncorrectAnswer" => 10,
];
$questions[] =
[
"leftAdder" => 16,
"rightAdder" => 32,
"correctAnswer" => 48,
"firstIncorrectAnswer" => 52,
"secondIncorrectAnswer" => 61,
];
$questions[] =
[
"leftAdder" => 45,
"rightAdder" => 12,
"correctAnswer" => 57,
"firstIncorrectAnswer" => 63,
"secondIncorrectAnswer" => 55,
];
$questions[] =
[
"leftAdder" => 42,
"rightAdder" => 18,
"correctAnswer" => 60,
"firstIncorrectAnswer" => 69,
"secondIncorrectAnswer" => 57
];
$questions[] =
[
"leftAdder" => 96,
"rightAdder" => 20,
"correctAnswer" => 116,
"firstIncorrectAnswer" => 120,
"secondIncorrectAnswer" => 110
];
$questions[] =
[
"leftAdder" => 44,
"rightAdder" => 85,
"correctAnswer" => 129,
"firstIncorrectAnswer" => 132,
"secondIncorrectAnswer" => 126
];
$questions[] =
[
"leftAdder" => 51,
"rightAdder" => 35,
"correctAnswer" => 86,
"firstIncorrectAnswer" => 96,
"secondIncorrectAnswer" => 82
];
$questions[] =
[
"leftAdder" => 5,
"rightAdder" => 61,
"correctAnswer" => 66,
"firstIncorrectAnswer" => 65,
"secondIncorrectAnswer" => 74
];
$questions[] =
[
"leftAdder" => 26,
"rightAdder" => 19,
"correctAnswer" => 45,
"firstIncorrectAnswer" => 40,
"secondIncorrectAnswer" => 39
];
$questions[] =
[
"leftAdder" => 26,
"rightAdder" => 35,
"correctAnswer" => 61,
"firstIncorrectAnswer" => 59,
"secondIncorrectAnswer" => 51
];
10 Answers
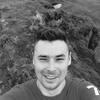
jamesjones21
9,260 Pointsyou can use a array_rand() on the array to output a different key from the array, but I would also do a for each loop on the array so that you get the key and value echo'd out.
How did you get onto the tech degree for php? Thought it was only front end ? As I asked the staff and they said there was no such course.
:)

Brandy Fishback
4,223 PointsThank you for offering help. I am brand new. I don't have a tech background. It is all so new to me but I am slowly getting there. I am still working on the quiz app. I have the array of 10 questions and answers. I am trying to get the app to show what number it is on like "question 2 of 10". Would I use a foreach loop?
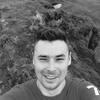
jamesjones21
9,260 Pointswhat you'll have to do is, put this into a form, then you can get your array, and put the array into a if statement, then if the answer is true, then move onto the next question like:
//question array
$questions = [
'question1' => ['What is my name?', 'James'],
'question2' => ['Where do I live?', 'UK']
];
$questionCount = 0;
//this is a multidimensional array the outer array key is named $question1, then the inner array is [0] = Question [1] = answer
if($question1[0][1] === 'James'){
echo 'correct';
//increments the question for each one they have right
$questionCount++;
} else {
echo 'wrong';
}
//the count then will increment up if correct, which you may use that to count

Brandy Fishback
4,223 PointsThank you so much for your help! I can't seem to get it to work. If you have time can you take a look at my code? https://github.com/BrandyLFishback/Project-Two
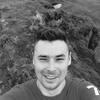
jamesjones21
9,260 PointsI can check for you sure, just will have to take a copy when I get home later on tonight, if this is ok?

Brandy Fishback
4,223 PointsThat's perfect! Thank you so much. I've pretty much butchered the project! The two things I've been working on is trying to get array Rand to work, right now only one of the questions is showing up. And then the counter.
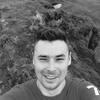
jamesjones21
9,260 PointsCan you create a new repository to store the files they provided?

Brandy Fishback
4,223 PointsSure! Here you go https://github.com/BrandyLFishback/Project-Two-Starter-Files
I really appreciate your help!

Brandy Fishback
4,223 PointsI think I just got array_rand to work!!! I am so happy. One of the requirements is to make sure the questions are not repeated until the question bank has been exhausted. I am not sure if that is happening yet.
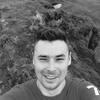
jamesjones21
9,260 Pointsthat's good, sorry didn't get on last night as been working flat out, how many projects do you get with this?

Brandy Fishback
4,223 PointsNo Problem! I worked until 9 pm last night and work 11 hours today. I won't even get to work on it until tomorrow. We get 9 projects and a capstone. There are 12 of us in this cohort. 6 in PHP and 6 in Java.
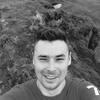
jamesjones21
9,260 PointsI've just built the random question generator in pure OOP PHP, so now spits out random questions and the correct answer etc

Brandy Fishback
4,223 PointsI would love to see your random quote generator!! Our random quote generator was very basic. The six girls in Java did it too but for this second project we have different projects.
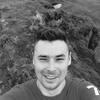
jamesjones21
9,260 Pointswas there any loops used to loop over the array ? I will put it up on my github sometime today.

Brandy Fishback
4,223 PointsNo their wasn't. Here is my random quote generator. I barley got it done. It was all so new to me. It is all starting to make sense to now:) https://github.com/BrandyLFishback/Project-1-Random-Quote-Generator/tree/master/a_random_quote_generator-v1
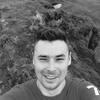
jamesjones21
9,260 Pointsthis is my OOP work in progress version:
RandomQuestion.php
<?php
// Generate random questions (Done)
// Loop for required number of questions
// Get random numbers to add (Done)
// Calculate correct answer
// Get incorrect answers within 10 numbers either way of correct answer
// Make sure it is a unique answer
// Add question and answer to questions array
include __DIR__ . '/Questions.php';
class RandomQuestions
{
public $count = 0;
public $used = [];
public function generateRandomQuestions()
{
$randomArray = Questions::questionHolder();
$rand = array_rand($randomArray, 1);
$send = $randomArray[$rand];
$this->count++;
return $this->displayQuestion($send);
}
public function displayQuestion($question)
{
$randomQuestion = $question;
$output = '<div>What is ' . $randomQuestion['leftAdder'] . ' + ' . $randomQuestion['rightAdder'] .'</div>';
$output .= '<button type="submit" name="correctAnswer" class="number">' . ($randomQuestion['leftAdder'] + $randomQuestion['rightAdder']) . '</button>';
$output .= '<button type="submit" name="firstIncorrectAnswer" class="number">'. $randomQuestion['firstIncorrectAnswer'] . '</button>';
$output .= '<button type="submit" name="secondIncorrectAnswer" class="number">'. $randomQuestion['secondIncorrectAnswer'] . '</button>';
return $output;
}
public function checkAnswer($correctAnswer, $firstWrong, $secondWrong)
{
$counter = $this->count;
$counter++;
}
}
$question = new RandomQuestions();
?>
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<title>Math Quiz Addition</title>
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" type="text/css" media="screen" href="main.css" />
</head>
<body>
<form action="<?php $_SERVER['PHP_SELF']; ?>" method="POST" class="form">
<label>Question <?= $question->count; ?> out of <?= Questions::numberQuestions(); ?></label>
<div class="content"></div>
<?= $question->generateRandomQuestions(); ?>
</div>
</form>
<script src="../main.js"></script>
</body>
</html>
Questions.php
<?php
class Questions
{
public static $correct = [];
public static function questionHolder()
{
$questions[] =
[
"leftAdder" => 3,
"rightAdder" => 4,
"correctAnswer" => 7,
"firstIncorrectAnswer" => 8,
"secondIncorrectAnswer" => 10,
];
$questions[] =
[
"leftAdder" => 16,
"rightAdder" => 32,
"correctAnswer" => 48,
"firstIncorrectAnswer" => 52,
"secondIncorrectAnswer" => 61,
];
$questions[] =
[
"leftAdder" => 45,
"rightAdder" => 12,
"correctAnswer" => 57,
"firstIncorrectAnswer" => 63,
"secondIncorrectAnswer" => 55,
];
$questions[] =
[
"leftAdder" => 42,
"rightAdder" => 18,
"correctAnswer" => 60,
"firstIncorrectAnswer" => 69,
"secondIncorrectAnswer" => 57
];
$questions[] =
[
"leftAdder" => 96,
"rightAdder" => 20,
"correctAnswer" => 116,
"firstIncorrectAnswer" => 120,
"secondIncorrectAnswer" => 110
];
$questions[] =
[
"leftAdder" => 44,
"rightAdder" => 85,
"correctAnswer" => 129,
"firstIncorrectAnswer" => 132,
"secondIncorrectAnswer" => 126
];
$questions[] =
[
"leftAdder" => 51,
"rightAdder" => 35,
"correctAnswer" => 86,
"firstIncorrectAnswer" => 96,
"secondIncorrectAnswer" => 82
];
$questions[] =
[
"leftAdder" => 5,
"rightAdder" => 61,
"correctAnswer" => 66,
"firstIncorrectAnswer" => 65,
"secondIncorrectAnswer" => 74
];
$questions[] =
[
"leftAdder" => 26,
"rightAdder" => 19,
"correctAnswer" => 45,
"firstIncorrectAnswer" => 40,
"secondIncorrectAnswer" => 39
];
$questions[] =
[
"leftAdder" => 26,
"rightAdder" => 35,
"correctAnswer" => 61,
"firstIncorrectAnswer" => 59,
"secondIncorrectAnswer" => 51
];
return $questions;
}
public static function numberQuestions()
{
return count(self::questionHolder());
}
}
So the code above generates a random question on each press, so all that is left to do is count the each correct question, randomise the answer buttons. which I do feel that this version will work best with a database as can't store answers lol Also the above comments at the very top is for the JS version?
hopefully you'll see how this works
Brandy Fishback
4,223 PointsBrandy Fishback
4,223 PointsThank you for your help! I was awarded a scholarship through a Talent Path program.
https://teamtreehouse.com/go/talentpath
jamesjones21
9,260 Pointsjamesjones21
9,260 Pointsthat is quite cool, if you are stuck with php do give me a shout I can lend a hand in it, be it procedural or OOP.