Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial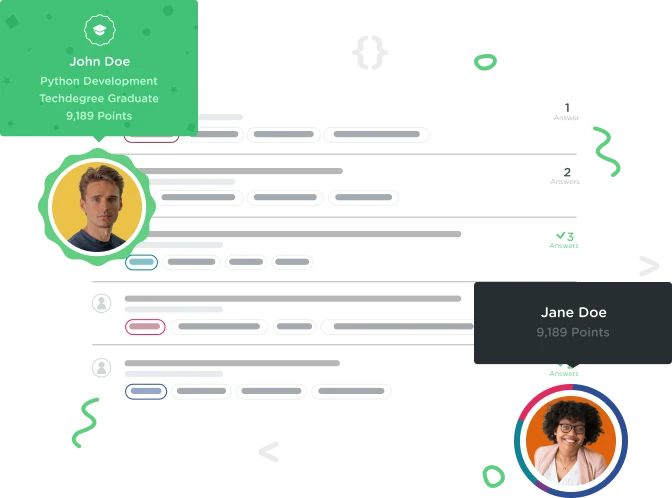
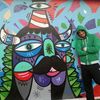
Vojislav Stupar
Courses Plus Student 12,217 PointsI have more dots than in the counter ???
After changing to for loop I wrote same code as in the solution , but after running the code I have 55 dots of different color. I changed to while loop and got the same number. if I change condition to 5 than I have 10 dots ???
var html = '';
var red;
var green;
var blue;
var rgbColor;
for (i=1; i<=10; i+=1 ) {
red = Math.floor(Math.random() * 256 );
green = Math.floor(Math.random() * 256 );
blue = Math.floor(Math.random() * 256 );
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
html += '<div style="background-color:' + rgbColor + '"></div>';
document.write(html);
}
Is there any logical answer ???
4 Answers

Nicholas Jensen
5,969 PointsIf you leave the concatenation of the html variable, AND write it to the document in the loop you multiply the number of dots you get. For instance, Loop 1 prints 1 dot Loop 2 prints 2 dots, total of 3 Loop 3 prints 3 dots, total of 6.
Perhaps it was a simple error in the programming? The code provided seemed to be pasted incorrectly?
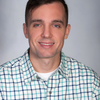
Jared Hensley
8,106 Pointsthis is correct.

ori
2,307 PointsDid you put the document.write(html); part inside or outside the loop?
When I tried the following code, it created the dots more times.
var html = '';
var red;
var green;
var blue;
var rgbColor;
for ( var i = 0; i < 10; i += 1) {
red = Math.floor(Math.random() * 256 );
green = Math.floor(Math.random() * 256 );
blue = Math.floor(Math.random() * 256 );
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
html += '<div style="background-color:' + rgbColor + '"></div>';
document.write(html);
}
When I removed the document.write(html); outside the loop, it worked fine.
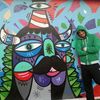
Vojislav Stupar
Courses Plus Student 12,217 PointsI think that was some bug in the workspace. Because when I used the same code using the workspace from the next video everything worked perfectly normal. There was 10 dots.
I think that document.write should be inside the loop because every time loop runs there are new random numbers generates for the rgb values and new dot is displayed on the screen.
There was simply 55 dots instead of 10 , after setting loop to run 5 times I head 10 dots on the screen like it should be. There is no logic in this ,simply there is some problem how is the browser interpreting the code. Code is fine it works. but not in the workspace related to the video itself.

Iain Simmons
Treehouse Moderator 32,305 PointsYour code was incorrect, and Nicholas Jensen and ori were correct, in that you were both adding to/updating the html
variable, and printing it multiple times instead of just the once at the end when it should contain the total/summed HTML from the loop.
55 instead of 10 and 10 instead of 5 is no coincidence, it's math:
1 + 2 + 3 + 4 + 5 + 6 + 7 + 8 + 9 + 10 = 55
1 + 2 + 3 + 4 + 5 = 10
You could also change your code so that you're completely replacing the contents of the html
variable in each iteration of the loop, and printing it to the screen before finishing that loop. i.e. replace html +=
with just html =
:
for ( var i = 0; i < 10; i += 1) {
...
html = '<div style="background-color:' + rgbColor + '"></div>';
document.write(html);
}
You should note however, that this will be less efficient, since it not only has to run the document.write
function multiple times instead of just once, but has to redraw
your HTML (almost like loading the HTML document again and again).
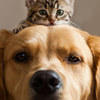
Devin Scheu
66,191 PointsTry setting the i variable in the for loop to 0, you might have more items because of that.
Vojislav Stupar
Courses Plus Student 12,217 PointsVojislav Stupar
Courses Plus Student 12,217 PointsOk I started workspace again from the next video and I wrote everything again. There is 10 dots as it should be. Weird. But now it's fine.