Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial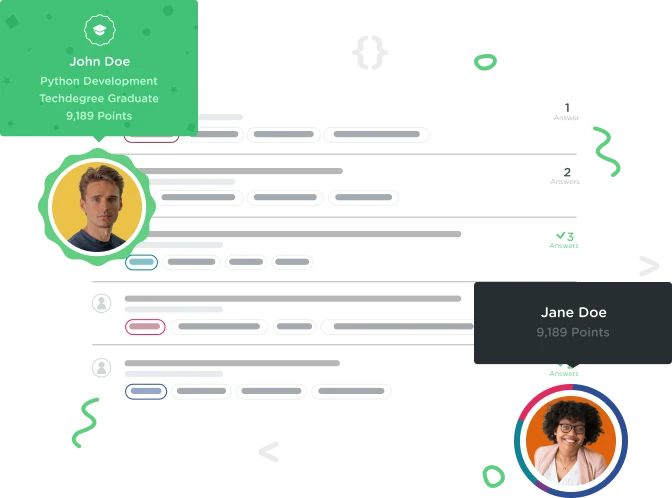

Pitrov Secondary
5,121 PointsI have no idea how to do this challenge
The only thing I know is that I need to do something with loops. But do I first have to split the string into multiple strings? But then how do I make those strings into dictionaries, and how can I count how many there are? Like I think I would have to make a long 25-50 lines of code to do it my way. How do I complete this challenge correctly?
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
word_count(sting):
3 Answers

Anthony Crespo
Python Web Development Techdegree Student 12,973 Pointsit's pretty much what you described and can be done in less than 10 lines
def word_count(arg="I do not like it Sam I Am"):
# here you lowercase every word in the string with .lower() and then split it with .split()
# it will return you a list object that look like this: ['i', 'do', 'not', 'like', 'it', 'sam', 'i', 'am']
words = arg.lower().split()
dictionnary = {}
for word in words:
# You check if the world is already in the dict
if word in dictionnary:
dictionnary[word] += 1 # increment the value if it is
# else you add it
else:
dictionnary[word] = 1
return dictionnary

Anthony Crespo
Python Web Development Techdegree Student 12,973 PointsNo problem buddy happy you got it. And if you like very very short code that look like a total mess you can do it in two lines.
def word_count(arg="I do not like it Sam I Am"):
return {word:arg.lower().split().count(word) for word in arg.lower().split()}

Pitrov Secondary
5,121 PointsWow, that's cool, I thought that it wasn't possible to make code shorter in python. Because you need indents after loops, if, elif and else.

Anthony Crespo
Python Web Development Techdegree Student 12,973 PointsYup! Python is pretty amazing and you can do a lot of stuff with a lot less code than a bunch of others languages.

Pitrov Secondary
5,121 PointsDid you learn to code here at Treehouse or did your school teach you to code?

Anthony Crespo
Python Web Development Techdegree Student 12,973 PointsOh no I am no expert and are still new to python and got a lot to learn! I started learning the basics of C++ and then found treehouse and now I love python. I got curious learning with treehouse and started to read a lot of docs and got the habit to google every single question I have.

Adam Paciorek
3,181 PointsHello All, I have solved it using the following code but the interpreter does not accept this solution. It seem to return the correct dictionary
def word_count(string):
#Lowercase the string
string = string.lower()
# Split on white space
list = string.split(" ")
#create a dictionary
dict = {}
#Insert key/value pairs
for item in list:
dict[item] = list.count(item)
# return dictionary
return dict
...not sure why the formatting of this code is off but I hope that you get my solution
Pitrov Secondary
5,121 PointsPitrov Secondary
5,121 PointsThanks! you really made it look simple. I like the way you explain.
Darrin Spell Jr
Full Stack JavaScript Techdegree Student 10,303 PointsDarrin Spell Jr
Full Stack JavaScript Techdegree Student 10,303 PointsI have a question. Why are you using arg in the parameter? I am confused on that part of the code.
arg = "I do not like it Sam I Am"
Anthony Crespo
Python Web Development Techdegree Student 12,973 PointsAnthony Crespo
Python Web Development Techdegree Student 12,973 Pointsarg="I do not like it Sam I Am" mean that the default value of arg is the string "I do not like it Sam I Am". You can write it like this instead so there is no default value:
def word_count(arg):
It was just to be more explicit on my example.