Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial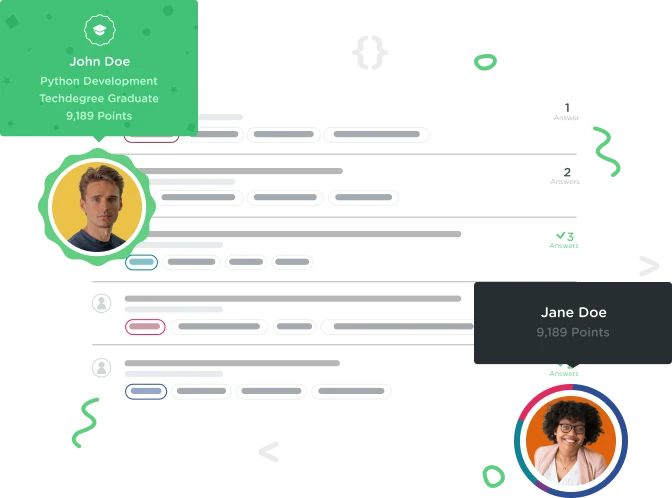
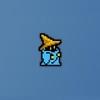
alekseibingham
4,491 PointsI have no idea were to even begin, Besides making the variable string_factory and setting it to take 1 argument, im lost
I have no idea on were to begin solving this besides defining the variable string_factory. I dont need the full blown answer, but i would like a explanation to the start of it, something to get me thinking i guess. I almost feel like im starting to lose my understanding with some of these newer coding challenges.
1 Answer

Ryan S
27,276 PointsHey Aleksei,
This challenge is a bit wordy and can seem like a lot at first. I find it helps to first figure out what the input and output is.
- Input: a list of dictionaries.
You are right that it will be a single argument, and as illustrated by the example in the challenge, each dictionary in the list will have two keys, each with a single value.
- Output: a new list, where each item is a formatted version of the
template
variable.
The template
variable has placeholders ("name", and "food"), which are the matching keys in the dictionaries.
So what you need to do is find a way to loop through each item in the list argument (each item being a dictionary), and unpack the values from that dictionary into the template
variable.
Hopefully this gets you started. Let me know if you need anymore assistance.
alekseibingham
4,491 Pointsalekseibingham
4,491 PointsSo, I have completed the challenge, so i thank you for taking your time to type me the above text. Although I used the internet to help research and attempt to understand different solutions to this probblem, here was my final code:
I dont quite understand it, I know the variable creates a blank list, then for each item found in the dictionary it adds it to the blank list starting with the template they gave us. But the **dic part I dont quite understand, if not at all. It was almost a wild guess, and another quick question, did i have to use "dict" as the argument for the function. Because upon typing it in, it highlighted its-self in another color, so im wondering if that was its own function of somesorts. Sorry if im not making since, im not picking up on dictionaries quite fast enough haha.
Also just figured out how to add code blocks to comments!
Ryan S
27,276 PointsRyan S
27,276 PointsThe way
**dic
is working in your code, is by matching placeholders in the string to the keys in the dictionary, and replacing the placeholders with the values in the dictionary.Here is a simple way to illustrate it using a single dictionary.
So what is happening is that the dictionary (my_dict) has matching keys to the placeholders in
template
. It is then unpacked with the ** operator and the values that belong to each key will be inserted in their proper place in the string.If the dictionary keys don't match the placeholders in
template
, you will get a KeyError.To answer your other question about the syntax highlighting, notice how
dict
is the same colour asfor
,return
, anddef
. This means that "dict" is a special keyword in Python and you should avoid using it as a variable name. It is like "list" or "tuple" and can be used to create a dictionary.Example:
In your code, it worked, but if you tried to use the "dict" keyword later in your function to create a dictionary, you won't be able to because you will have overwritten it as a variable.
This is one place where syntax highlighting is really handy, especially when learning a new language, because you may stumble upon keywords you didn't know existed.
Hope this clears things up.
Patricia Snyder
5,563 PointsPatricia Snyder
5,563 PointsHi, I have a question about the finished code. I understand most of it, but I'm still really confused as to where to put the *kwarg. Why is the * on dic instead of **dict?
Thank you!
Ryan S
27,276 PointsRyan S
27,276 PointsHey Patricia,
You bring up a good question, and in the future I would recommend posting a new question in the forum, that way it'll be easier for others to find, since many will likely have the same question.
But to answer it, the reason we don't use **kwargs as the input parameter is because we know that there will only be one input argument, and that argument is a
list
. Using **kwargs would allow us to pass in an unknown amount of keyword arguments to the function, but that is not needed here because we know it will be just one list.This list has an unknown amount of items, but each item happens to be a dictionary, And each dictionary will have 2 keys (name and food).
So when we are iterating through each item in the list, "
dic
" represents a single dictionary. We unpack this dictionary in the.format()
method in order to match the values in the dictionary with the keywords in the template string.Also, one other thing is that you should avoid using the word "dict" as a variable name, since this is already a Python type class, just like 'list' or 'int'. If you tried to create a dictionary using the "dict" keyword later in your code, you would get an error since you have overwritten it. Usually a text editor will highlight reserved words and keywords for you so you can visually tell if you accidentally used one you didn't know about.
Hope this clears things up.
Patricia Snyder
5,563 PointsPatricia Snyder
5,563 PointsYes, thank you.