Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial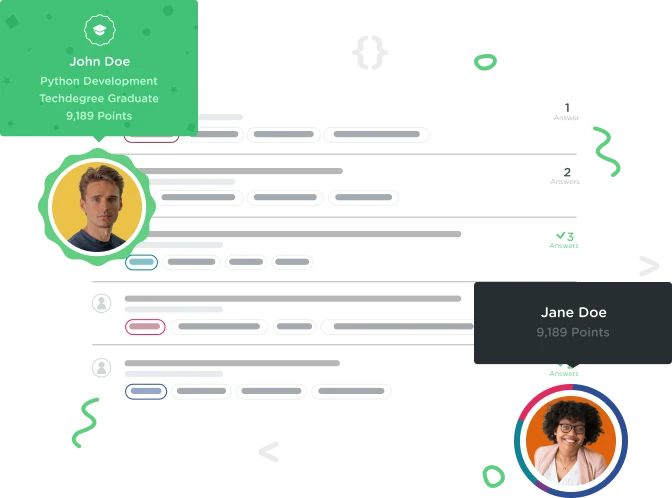

David Hsu
2,574 PointsI have some confusion around using a for loop and remove function
I was trying to solve this using a for loop and remove, and it seems when it did this, the for loop skipped the next item in the list after the previous item was removed.
my code:
the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
for item in the_list:
print("evaluating {}, {}".format(item,type(item)))
if type(item) is str:
the_list.remove(item)
elif type(item) is bool:
the_list.remove(item)
elif type(item) is list:
the_list.remove(item)
else:
print "continuing"
print the_list
the output while debugging:
evaluating a, <type 'str'>
[2, 3, 1, False, [1, 2, 3]]
evaluating 3, <type 'int'>
continuing
[2, 3, 1, False, [1, 2, 3]]
evaluating 1, <type 'int'>
continuing
[2, 3, 1, False, [1, 2, 3]]
evaluating False, <type 'bool'>
[2, 3, 1, [1, 2, 3]]
so after "a" is removed, the next time in the for loop, it seemed to skip over evaluating 2. The same happened when it removed False; it never evaluates [1,2,3] as a list.
is the generated list of things in the for loop static, so remove() is making the next item in the list skipped?
2 Answers

David Hsu
2,574 Pointsafter poking around, I learned about the reversed() function for the for loop. If I did:
the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
for item in reversed(the_list):
print("evaluating {}, {}".format(item,type(item)))
if type(item) is str:
the_list.remove(item)
elif type(item) is bool:
the_list.remove(item)
elif type(item) is list:
the_list.remove(item)
else:
print "continuing"
print the_list
then it functioned as I wanted. I could have made a copy of the_list to manipulate, and that would have worked as well.

David Hsu
2,574 PointsI have it figured out, thanks.
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsHi David,
Do you still need an explanation of why your original code didn't work or do you think you have it figured out?