Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial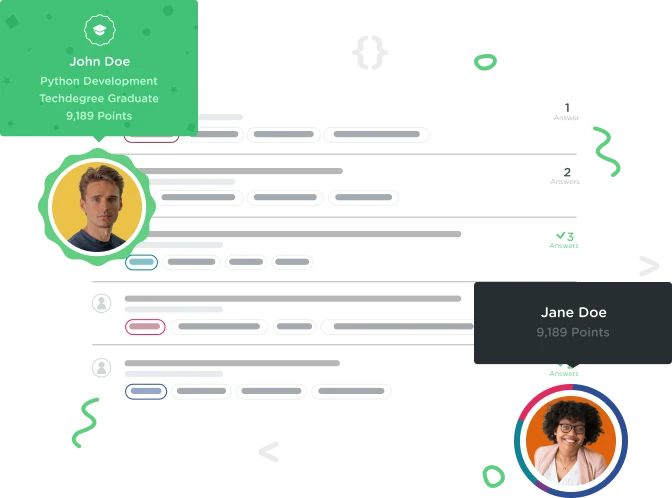

Sophie King
Full Stack JavaScript Techdegree Student 1,685 PointsI have tried to include as much as possible of what we have learnt so far. Could my Q5 be tidier?
// Simple quizz game
var score = 0;
// Question 1
var question1 = prompt('Hi, welcome to the quiz! \n Q1. What color is grass?');
if (question1.toUpperCase() === 'GREEN' ) {
score ++;
alert('correct! Your score is ' + score + ' out of 5');
} else {
alert('Incorrect! Your score is ' + score + ' out of 5');
}
// Question 2
var question2 = prompt('Next question! \n Q2. Can you write a number that is more than 10, but less than 20?');
if (parseInt(question2) > 10 && parseInt(question2) < 20 ) {
score ++;
alert('correct! Your score is ' + score + ' out of 5');
} else {
alert('Incorrect! Your score is ' + score + ' out of 5');
}
// Question 3
var question3 = prompt('Next question! \n Q3. How many days are there in a week?');
if (question3.toUpperCase() === 'SEVEN' || parseInt(question3) === 7) {
score ++;
alert('correct! Your score is ' + score + ' out of 5');
} else {
alert('Incorrect! Your score is ' + score + ' out of 5');
}
// Question 4
var question4 = prompt('Next question! \n Q4. Name a month of the year beginning with "J" ');
if ( question4.toUpperCase() === 'JANUARY' || question4.toUpperCase() === 'JUNE' || question4.toUpperCase() === 'JULY' ) {
score ++;
alert('correct! Your score is ' + score + ' out of 5');
} else {
alert('Incorrect! Your score is ' + score + ' out of 5');
}
// Question 5
var question5 = prompt('Ok, last question! \n Q5. Pick any number');
var part2 = prompt('Times your number (' + question5 + ') by 2 and type the answer below.');
var doubleNumber = question5 * 2;
if (part2 === question5 * 2) {
score ++;
alert('correct! Your score is ' + score + ' out of 5');
} else {
alert('Incorrect! Your score is ' + score + ' out of 5');
}
//Display final score
document.write('<h1> Your final score is ' + score + ' out of 5 </h1>');
2 Answers

Andrew Hinkson
Full Stack JavaScript Techdegree Graduate 25,822 PointsLooking great! The only issue I see is that prompt for question 5 the number comes back as a string so when you declare doubleNumber you could:
var doubleNumber = parseInt(question5) * 5;
Then in the if condition use doubleNumber :
if(part2 === doubleNumber) {...}
The parseInt() takes the number the use inputs into the prompt and turns it into a integer that can be multiplied.

Sophie King
Full Stack JavaScript Techdegree Student 1,685 PointsThat's really helpful, thank you! I did try the first way round, but it wouldn't accept any answers as correct.

Andrew Hinkson
Full Stack JavaScript Techdegree Graduate 25,822 PointsYea its all about the fact that prompt returns strings so you would have to make sure to parseInt() anthing that comes from there thats an integer.
Im glad I could help.
Andrew Hinkson
Full Stack JavaScript Techdegree Graduate 25,822 PointsAndrew Hinkson
Full Stack JavaScript Techdegree Graduate 25,822 Pointsand actually you will need to use parseInt() on part2 also just to make sure the if statements conditions work correctly so you would also change this:
That way both part2 and doubleNumber are both integers and they will compare correctly.
Sorry this is a bit much possibly but hopefully it helps.