Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial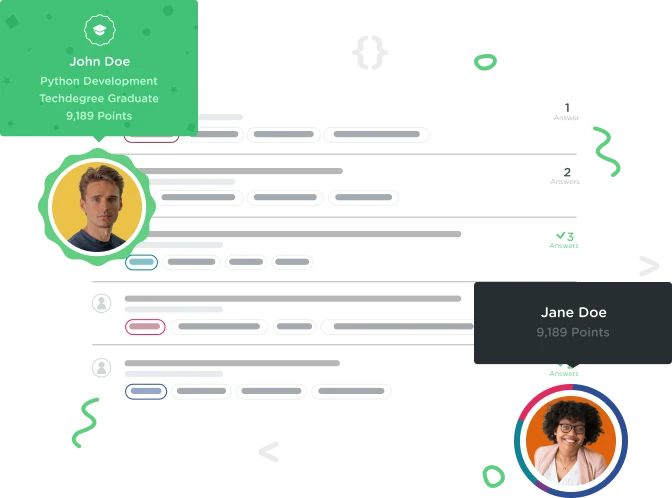

Jakob Hyldgaard
9,566 Pointsi have typed this the answer and have tried it in python idle 3.5.2 shell. but treehouse says its not correct.
//here is my answer
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(string):
words = {}
string = string.lower()
while " " in string:
index = string.find(" ")
if string[:index] in words:
words[string[:index]] = words[string[:index]]+1
else:
words[string[:index]] = 1
string = list(string)
del string[:index+1]
string = "".join(string)
if string[:] in words:
words[string[:]] = words[string[:]]+1
else:
words[string[:]] = 1
return(words)
2 Answers
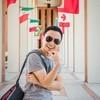
hamsternation
26,616 PointsHello!
Your method is genius! Took me a second to figure out what it did and why it works! :)
There's a tiny issue with your method, though, and that's when there's a trailing space at the end of the string, your code adds an excess dictionary item to the end of the dictionary. Try it out and you'll see.
I'm guessing that's why it invalidates your code.
The test is most likely looking for an alternate method involving the .split method to create a list. This method splits by the spaces and ignores the trailing spaces at the end of the sentences.
:) good luck!
edit Actually, no I take that back... split adds the spaces to the dict as well. In that case, this challenge must be looking specifically for the .split method then... I forgot how I passed it but the .split method should get you through.

Jakob Hyldgaard
9,566 PointsHello!
Thank you for your fast response.
I have not heard of the split() method before, but have researched a bit and changed my code. I have found a way to drop the trailing space and have tried both with or without it and i still can't manage to complete the challenge.
I have tried again with the new code but i keep getting the same error as before:
"Hmm, didn't get the expected output. Be sure you're lowercasing the string and splitting on all whitespace!"
Well anyway thank you for your great response and even though i don't know how to get through this challenge it helped me a lot in my learning.
btw this is the new code that i have tried:
code
def word_count(string):
string = string.lower()
word = {}
word_list = string.split(" ")
for words in word_list:
if words == "":
pass
elif words in word:
word[words] = word[words]+1
else:
word[words] = 1
return(word)