Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial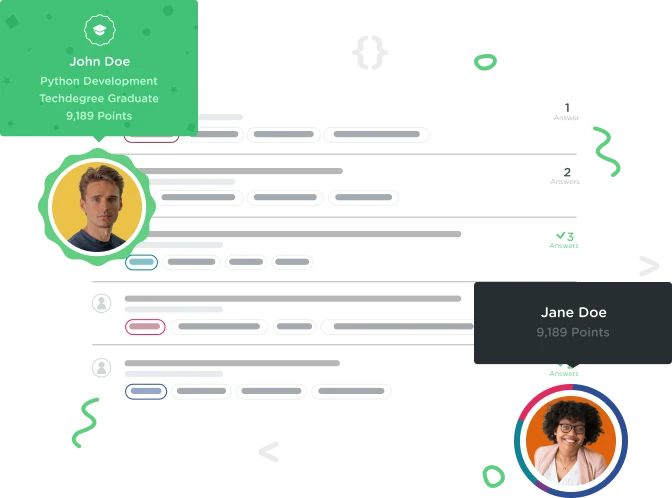

Brandon Harrison
1,283 PointsI keep getting an error saying I am not returning the right thing
Question:
"Write a function named squared that takes a single argument. If the argument can be converted into an integer, convert it and return the square of the number (num ** 2 or num * num). If the argument cannot be turned into an integer (maybe it's a string of non-numbers?), return the argument multiplied by its length. Look in the file for examples."
This has me completely stumped and I feel like I have approached it a million ways. What am I doing wrong?
# EXAMPLES
# squared(5) would return 25
# squared("2") would return 4
# squared("tim") would return "timtimtim"
def squared(i):
if i == int:
return i ** 2
if i == str:
return str(i) * len()
1 Answer

Sahil Sharma
4,791 PointsIf you want to check if a variable has a number value then use
if str(i).isdigit():
return ( i ** 2)
else:
return (str(i) * len(i))
notice that I used len(i), you have to pass the argument of the iterable whose length you want in the parenthesis. Apart from this you can also try an alternative approach by using try and except. try to convert the value stored in i to an int using
try:
i = int(i)
except ValueError:
return (str(i) * len(i))
else:
return (i * i)
Brandon Harrison
1,283 PointsBrandon Harrison
1,283 PointsThank you so much. The try block worked and I had to read it a couple of times for it to make sense to me but now I got it.
Sahil Sharma
4,791 PointsSahil Sharma
4,791 PointsOops, I forgot to add
i = int(i)
in the if block statement as otherwise return(i ** 2) will throw an error.