Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial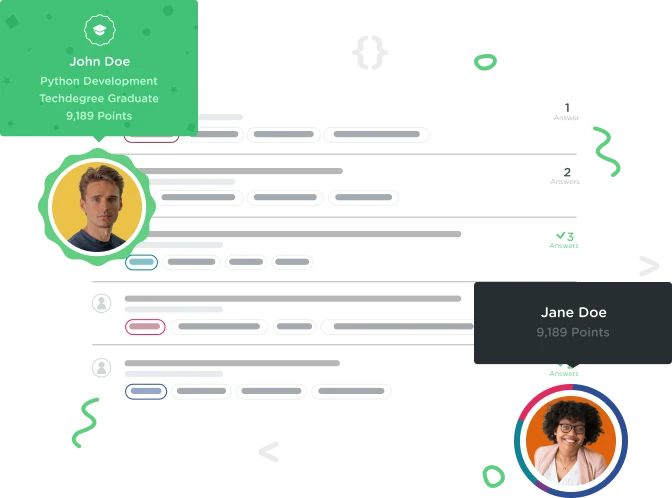
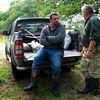
mark gilchrist
Courses Plus Student 33,169 PointsI keep getting "wrong number of prints" and there is nothing in the output, How do you debug this?
I am lost as to how you debug this, please help
import random
start = 5
while start <0:
i = random.randint(1,99)
if even_odd(i) :
print("{} is even")
else :
print("{} is odd".format(i))
start = start - 1
def even_odd(num):
return not num % 2
2 Answers

Jeff Wilton
16,646 PointsI found 3 issues with your code (not bad really - you were rather close).
1: your even_odd method needs to be defined before trying to call it.
2: you accidentally had a less than symbol in your while statement.
3: your forgot to pass in the i in the string formatter in your first print statement.
import random
start = 5
def even_odd(num):
return not num % 2
while start > 0:
i = random.randint(1,99)
if even_odd(i) :
print("{} is even".format(i))
else :
print("{} is odd".format(i))
start = start - 1
Sometimes the error messages they give you don't really explain what the problem is. In this case it was easy enough to step through your code and see the issues, but for heavier debugging don't be afraid to paste your code into a python shell in your local system (or even workspaces) and try to run it. For future debugging references, you can create a breakpoint anywhere in your code with this statement:
import pdb; pdb.set_trace()
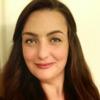
Jennifer Nordell
Treehouse TeacherThere are two errors in your code. First, the even_odd function has to appear in the code before it's called. Otherwise it doesn't know what that function is. As I see you have some JavaScript knowledge it's important to note that in JavaScript you can declare a function elsewhere in your code. This is not the case here.
Secondly, your while loop will never run. It's set to run while the start value is less than 0. It's initialized at 5. So at the first time it comes to the while loop... 5 is not less than 0 and thus never runs. You simply reversed the greater than and less than sign.
Take a look at your edited code that will pass the challenge:
import random
start = 5
def even_odd(num):
return not num % 2
while start > 0:
i = random.randint(1,99)
if even_odd(i) :
print("{} is even")
else :
print("{} is odd".format(i))
start = start - 1
Jennifer Nordell
Treehouse TeacherJennifer Nordell
Treehouse TeacherGreat catch with that missing i variable that wasn't passed into the string formatting. I completely missed that one. The code I posted shouldn't pass then... but it does :/